How to Perform SERP Scraping in Python A Deep Dive
How to perform SERP scraping in Python is a powerful technique for data extraction from search engine results pages (SERPs). This guide delves into the process, from fundamental concepts to advanced techniques, ensuring ethical and legal compliance. We’ll explore various Python libraries, data handling strategies, and crucial considerations for building robust and scalable scraping solutions.
This comprehensive guide will walk you through the entire process of SERP scraping, from understanding the ethical implications and legal limitations to leveraging the most effective Python libraries and handling diverse data formats. We’ll cover everything from installing libraries and fetching web pages to extracting specific data elements, handling dynamic content, and storing the collected data securely.
Introduction to SERP Scraping in Python
SERP scraping, or Search Engine Results Page scraping, involves automatically extracting data from search engine results pages (SERPs). This data can encompass various elements like titles, descriptions, URLs, and even image previews, providing valuable insights into search results, competitor analysis, and research. A crucial aspect is understanding the ethical and legal boundaries of this practice.This process, while potentially powerful, comes with significant ethical and legal considerations.
It’s vital to respect website terms of service and robots.txt files, as unauthorized data collection can lead to legal repercussions and harm website owners. Python’s powerful libraries make web scraping feasible, but ethical considerations must always guide the process.
Ethical Considerations and Legal Limitations
Web scraping, while seemingly innocuous, can have significant ethical and legal implications. Respecting website terms of service is paramount. Many websites explicitly prohibit automated data collection, and violating these terms can result in legal issues. Similarly, robots.txt files are crucial. These files dictate which parts of a website’s content are accessible to crawlers.
Ignoring these directives can overload servers and disrupt the website’s operation.
Fundamental Concepts of Web Scraping in Python
Python’s rich ecosystem of libraries facilitates web scraping. Libraries like `requests` are used to fetch web pages, while `BeautifulSoup` parses the HTML or XML content. These tools allow you to extract specific information from the retrieved pages. This process involves understanding HTML and CSS structures to pinpoint the data you need.
Respecting Robots.txt and Website Terms of Service
Before initiating any web scraping operation, meticulously review the robots.txt file for the target website. This file Artikels which parts of the site are accessible to automated bots. By adhering to these guidelines, you prevent overloading the website’s servers and maintain a positive relationship with the site owners. Understanding and respecting the website’s terms of service is equally important.
Review the terms of service for any explicit prohibitions on automated data collection. This proactive approach avoids legal issues and promotes ethical web scraping practices.
Comparison of Web Scraping Methods
Different methods offer varying levels of efficiency and control. One common method involves using libraries like `requests` and `BeautifulSoup` to directly parse HTML content. Another approach involves using dedicated scraping tools or frameworks. These frameworks often provide more advanced features, but they might be overkill for simple tasks. The selection of a method should be based on the complexity of the task and the desired level of control.
Method | Description | Advantages | Disadvantages |
---|---|---|---|
Direct Parsing | Using libraries like `requests` and `BeautifulSoup` to parse HTML directly. | Simple to implement for basic tasks. | Less robust for complex sites. |
Dedicated Scraping Tools/Frameworks | Specialized tools offering features like handling complex websites and avoiding rate limits. | More robust and efficient for complex sites. | Steeper learning curve and potentially more expensive. |
Libraries for SERP Scraping in Python: How To Perform Serp Scraping In Python
SERP scraping, the process of extracting data from search engine results pages (SERPs), requires robust and efficient Python libraries. These tools automate the retrieval of information, enabling tasks like competitive analysis, research, and trend monitoring. Choosing the right library is crucial for successful scraping, as different libraries excel in different areas.
Common Python Libraries for SERP Scraping
Several Python libraries facilitate SERP scraping. Popular choices include Beautiful Soup, Scrapy, and Selenium. Each library offers unique strengths and weaknesses, impacting scraping efficiency and the types of tasks they are best suited for.
Beautiful Soup
Beautiful Soup is a widely used Python library for parsing HTML and XML documents. It’s particularly valuable for extracting structured data from web pages. Beautiful Soup excels at handling messy or inconsistently formatted HTML, making it suitable for scraping SERPs where the structure isn’t always predictable. A key advantage is its relative simplicity, making it easier for beginners to get started.
Strengths: Excellent for parsing HTML and XML, handles messy data well, straightforward to learn.
Weaknesses: Not ideal for dynamic websites or those using JavaScript for rendering content, can be slower for large-scale scraping.
Example:
from bs4 import BeautifulSoup
import requests
# Fetch the SERP page
response = requests.get("https://www.google.com/search?q=python+scraping")
soup = BeautifulSoup(response.content, "html.parser")
# Extract relevant data (example: title of the first result)
title = soup.find("h3", class_="LC20lb").text
print(title)
Scrapy
Scrapy is a powerful, open-source framework designed for web scraping. It’s more complex than Beautiful Soup, but it offers significantly more advanced features, making it suitable for large-scale and complex scraping projects. Scrapy excels at handling multiple requests, allowing for parallel processing, which boosts scraping speed.
Strengths: Robust framework for large-scale scraping, supports parallel processing for speed, extensive features for handling different scraping scenarios.
Weaknesses: Steeper learning curve compared to Beautiful Soup, more complex to set up for simple tasks.
Example:
# (Example using Scrapy - a more complex setup is needed for this framework)
# ... Scrapy setup and spider definition ...
# ... extract relevant data from the response
Selenium
Selenium is a browser automation tool, enabling interaction with websites as a user would. This is particularly useful for scraping dynamic websites that load content using JavaScript. Selenium can handle situations where content isn’t present in the initial HTML source. However, it can be slower than other libraries due to the browser interaction.
Strengths: Handles dynamic content effectively, simulates user interaction with the website, suitable for sites with JavaScript rendering.
Weaknesses: Can be significantly slower than other libraries, requires installing and configuring a web browser driver.
Example:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize a WebDriver (e.g., Chrome)
driver = webdriver.Chrome()
driver.get("https://www.example.com")
# ... Find elements and extract data ...
driver.quit()
Library Comparison
Library | Features | Ease of Use | Pros |
---|---|---|---|
Beautiful Soup | HTML/XML parsing | Easy | Simple to learn, handles messy data |
Scrapy | Large-scale scraping, parallel processing | Moderate | Robust, high performance for large projects |
Selenium | Dynamic content, browser interaction | Moderate | Handles JavaScript-heavy sites, simulates user behavior |
Handling Web Pages and Extracting Data
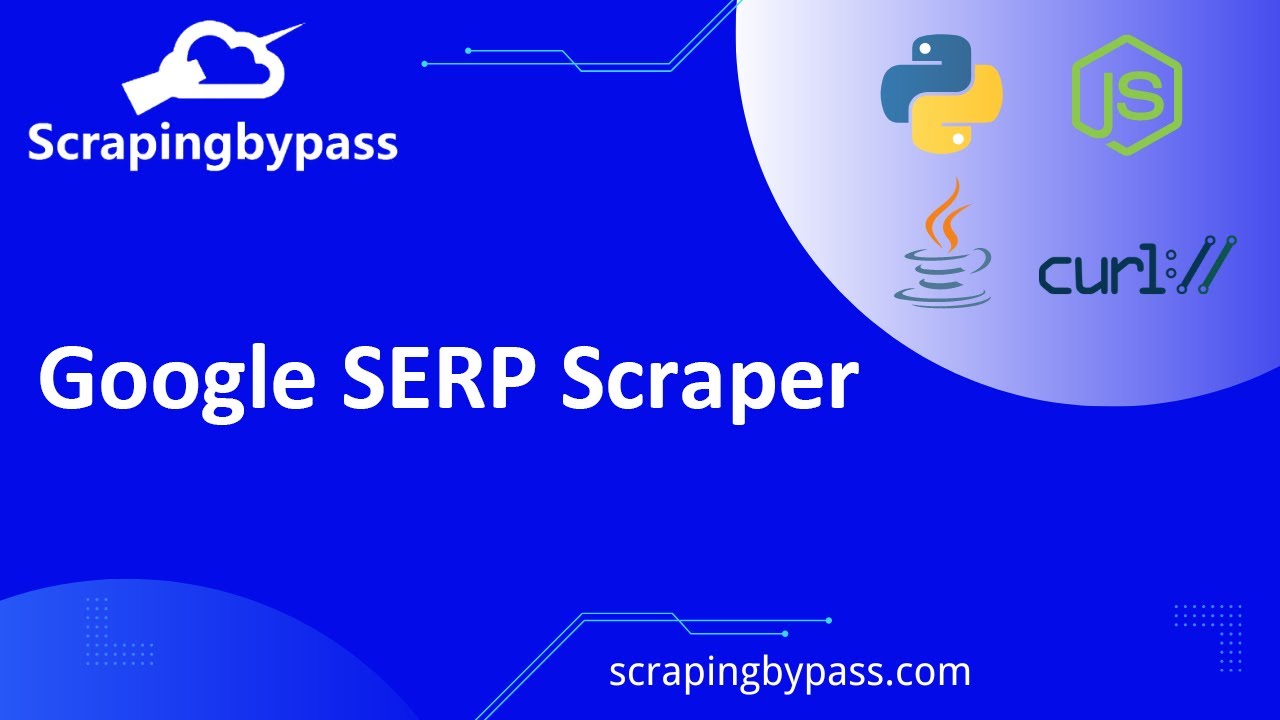
Fetching and parsing web pages is crucial for SERP scraping. Understanding how to efficiently retrieve and process the HTML structure allows you to extract the desired data points from search engine results pages (SERPs). This involves navigating the complexities of web pages, handling dynamic content, and ensuring robustness in the face of varying HTML structures.
Effective web page handling is paramount in SERP scraping. The process involves a sequence of steps, from fetching the page to extracting the necessary information. Accurate data extraction depends on understanding the structure and format of the web pages, and robust strategies are essential to cope with the variability of data sources.
Fetching Web Pages
Python offers powerful libraries like `requests` for fetching web pages. `requests` simplifies the process of making HTTP requests to retrieve the HTML content of a webpage. This step is fundamental to any scraping operation. The `requests` library handles headers, cookies, and other important aspects of web communication, making it a reliable choice for web page retrieval.
“`python
import requests
url = “https://www.example.com”
response = requests.get(url)
response.status_code # Check for successful retrieval
html_content = response.content
“`
This example demonstrates how to fetch a webpage using `requests`. The code first imports the `requests` library, then defines the URL of the target page. It uses `requests.get()` to retrieve the page’s content. Checking the `response.status_code` ensures a successful request. Finally, `response.content` gives you the raw HTML content.
Parsing HTML
Parsing HTML involves transforming the raw HTML into a structured format that allows easy data extraction. Libraries like `BeautifulSoup` are widely used for this task. `BeautifulSoup` converts the raw HTML into a tree-like structure, enabling you to traverse the elements and extract information based on tags, attributes, and other properties.
“`python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, ‘html.parser’)
“`
This example uses `BeautifulSoup` to parse the HTML content. The `BeautifulSoup` object allows you to search for elements using methods like `find()` and `find_all()`.
Extracting Relevant Data
Identifying and extracting the specific data elements from the parsed HTML is crucial. This involves targeting the relevant tags, attributes, or text content within the HTML structure. Methods like `find()` and `find_all()` within `BeautifulSoup` help locate specific elements.
“`python
title_element = soup.find(‘title’)
title_text = title_element.text if title_element else None
“`
This code snippet illustrates extracting the title of a web page. It searches for the `
Handling Dynamic Content
Many websites use JavaScript to render content dynamically. This presents a challenge for scraping, as the initial HTML might not contain the desired data. Approaches such as using tools like Selenium can render the page fully, enabling accurate data extraction.
Selenium automates a web browser, allowing you to interact with the page and execute JavaScript code, making it useful for handling dynamic content.
Efficient Extraction
Crafting methods for efficiently extracting specific elements is vital for SERP scraping. This involves understanding the HTML structure and employing efficient search strategies. Using CSS selectors can improve the efficiency and maintainability of your extraction process. They allow for more precise targeting of elements.
“`python
import requests
from bs4 import BeautifulSoup
url = “https://www.example.com”
response = requests.get(url)
soup = BeautifulSoup(response.content, ‘html.parser’)
# Use CSS selectors for efficient element targeting
results = soup.select(‘div.result’) # Example: finding elements with class “result”
“`
Handling Different HTML Structures and Data Formats
Web pages can have diverse HTML structures and data formats. Scraping strategies should be adaptable to these variations. This involves using robust parsing techniques to handle different tag structures, attributes, and content formats.
Data Handling and Storage
Once you’ve successfully extracted the SERP data, the next crucial step is to handle and store it effectively. This involves choosing the right format, dealing with potential data inconsistencies, and designing a robust storage mechanism. Efficient data management ensures that your extracted insights are accessible and usable for further analysis and reporting.
Storing extracted SERP data in a structured and manageable format is paramount. This allows for easy retrieval, analysis, and manipulation of the information. Various methods and formats exist for achieving this, ranging from simple CSV files to complex relational databases.
Methods for Storing Extracted Data
Various methods can be employed to store extracted data, catering to different needs and project scales. Choosing the right method depends on the volume of data, the desired level of organization, and the intended use cases.
- File-based storage: Simple formats like CSV and JSON files are suitable for smaller datasets. These formats are straightforward to read and write, making them easy to integrate with Python scripts. For instance, CSV files are perfect for tabular data, while JSON is ideal for structured data with key-value pairs. CSV files are well-suited for quick data dumps, while JSON files provide better structure and readability for more complex data.
Tools like Pandas in Python excel at working with CSV files, while libraries like `json` can handle JSON files efficiently.
- Database storage: For larger datasets and more complex analyses, relational databases like PostgreSQL, MySQL, or SQLite offer significant advantages. Databases provide structured storage, enabling efficient querying and data manipulation. Database storage ensures data integrity and scalability, becoming increasingly essential as the amount of data increases.
Different Data Formats
Choosing the right data format is crucial for efficient storage and retrieval. The selection depends on the nature of the extracted data and the intended use cases.
Learning how to perform SERP scraping in Python is a cool skill, but sometimes it’s nice to take a break and see what’s happening in the celeb world. Like, did you see how John Mulaney roasted Meghan Markle and Prince Harry at the star-studded Netflix event? Here’s the scoop. Anyway, back to Python, you’ll need libraries like Beautiful Soup and Requests to effectively pull data from search results.
- CSV (Comma-Separated Values): A simple text-based format suitable for tabular data. It’s easily readable by humans and can be processed by various tools and programming languages. CSV files are commonly used for exporting and importing data from spreadsheets.
- JSON (JavaScript Object Notation): A lightweight data-interchange format that’s ideal for structured data. It uses key-value pairs and nested structures, making it suitable for representing complex data hierarchies. JSON is widely used in web applications and APIs.
- Parquet: A columnar storage format designed for efficient data querying and analysis. It compresses data and optimizes storage space, especially beneficial for large datasets. Parquet is commonly used in data warehousing and analytics.
Handling Large Datasets
Handling massive datasets requires careful consideration of storage and processing strategies. Optimizing storage and processing becomes essential to prevent performance bottlenecks.
- Chunking: Break down the data into smaller, manageable chunks for processing. This approach is particularly helpful for large files or datasets that cannot fit entirely into memory. Iterating through the data in smaller portions significantly improves efficiency.
- Data Compression: Techniques like gzip or bz2 compression can reduce the size of data files, making storage and retrieval more efficient. This is especially valuable for very large datasets.
- Database Optimization: For database storage, using appropriate indexing and query optimization strategies can significantly improve retrieval speed. Indexing allows for faster data retrieval, which is crucial for large datasets.
Storing Extracted Data in a Database
Databases provide structured storage for extracted data, offering efficient querying and manipulation.
“`python
import sqlite3
# Sample connection to a SQLite database
conn = sqlite3.connect(‘serp_data.db’)
cursor = conn.cursor()
# Create a table to store the data
cursor.execute(”’
CREATE TABLE IF NOT EXISTS search_results (
query TEXT,
position INTEGER,
title TEXT,
url TEXT
)
”’)
# Sample data (replace with your extracted data)
data = [
(‘python scraping tutorial’, 1, ‘Python Scraping Tutorial – A Beginner\’s Guide’, ‘https://www.example.com/tutorial’),
(‘web scraping tutorial’, 2, ‘Web Scraping Tutorial – Advanced Techniques’, ‘https://www.example.com/advanced’)
]
# Insert data into the table
for query, position, title, url in data:
cursor.execute(“INSERT INTO search_results VALUES (?, ?, ?, ?)”, (query, position, title, url))
conn.commit()
conn.close()
“`
This code snippet demonstrates creating a SQLite database and inserting data into a table. Adapt the table structure and data insertion logic to fit your specific extraction needs.
Data Cleaning and Preprocessing Techniques
Data cleaning and preprocessing are crucial steps in preparing the extracted data for analysis. Inconsistencies, errors, and irrelevant information can significantly affect the quality of insights.
- Handling Missing Values: Missing values (NaN or None) need to be addressed. Strategies include imputation (filling with a calculated value) or removal (deleting rows with missing data).
- Data Transformation: Convert data types as needed (e.g., string to integer). This step is crucial for performing calculations or comparisons on the data.
- Duplicate Removal: Identify and remove duplicate entries to avoid redundancy in analysis.
- Text Preprocessing (for textual data): Clean and standardize text data to improve analysis accuracy. This might involve removing special characters, converting to lowercase, and stemming or lemmatizing words.
Implementing Robust and Scalable Scraping
Robust and scalable scraping is crucial for maintaining a reliable data pipeline. This involves techniques to avoid getting blocked by websites, handling rate limits, and effectively managing errors. This ensures data collection is continuous and efficient, allowing for consistent updates and analysis.
Learning how to perform SERP scraping in Python can be a fun and useful skill, especially when you’re looking for data on trending topics. For example, you might be interested in the recent news about Mexico City banning violent bullfighting, which is causing a lot of controversy, as reported in this article mexico city bans violent bullfighting sparking fury and celebration.
Once you master the basics of Python libraries like Beautiful Soup and Requests, you can easily extract relevant information from search engine results pages (SERPs), which can then be used for analysis or other projects.
Effective scraping requires a deep understanding of web server behavior and the protocols it uses. Ignoring these factors can lead to your scraper being identified as a threat and blocked. This document will Artikel strategies to prevent these issues and create a resilient data acquisition system.
Strategies for Avoiding Website Blocks
Websites employ various methods to detect and prevent malicious or excessive scraping activity. Recognizing and adhering to robots.txt files is essential. These files specify which parts of a website should not be indexed by bots, including scrapers. Thorough review and respect for these instructions can prevent accidental violations. Following the site’s terms of service is paramount, as violations often result in IP blocking.
Using a consistent user agent and avoiding rapid requests can also reduce the risk of being flagged as a bot.
Techniques for Handling Rate Limiting
Rate limiting is a common defense mechanism against excessive requests. Websites impose limits on the number of requests a single user or IP address can make within a specific timeframe. To overcome this, implement delays between requests. This allows the website’s servers time to process requests without overwhelming them. Using libraries like `time.sleep()` can introduce delays to avoid triggering rate limits.
Alternatively, consider using a dedicated scraping library that handles rate limiting automatically.
Methods for Handling Errors and Exceptions During Scraping
Scraping involves numerous potential errors and exceptions. Handling these issues gracefully is essential to prevent the scraper from crashing or failing. Implementing robust error handling with `try-except` blocks is critical. This approach allows the scraper to catch and manage errors such as connection timeouts, HTTP errors (like 404 Not Found), or invalid data formats. Logging these errors is essential for debugging and identifying patterns in the scraping process.
Implementing Delays Between Requests
Introducing delays between requests is a fundamental technique for avoiding rate limiting. This allows the website’s servers time to process requests without overwhelming them. Use the `time.sleep()` function to introduce a specified delay between each request. The duration of the delay should be determined by the website’s rate limits.
Handling Different Response Codes
Different HTTP response codes indicate various conditions during a request. Understanding these codes is vital for troubleshooting and error handling. Common response codes like 200 (OK) indicate successful requests, while 4xx (Client Error) and 5xx (Server Error) codes indicate issues. The scraper should handle these codes appropriately, logging the errors and potentially retrying the request after a delay.
Advanced Techniques for SERP Scraping
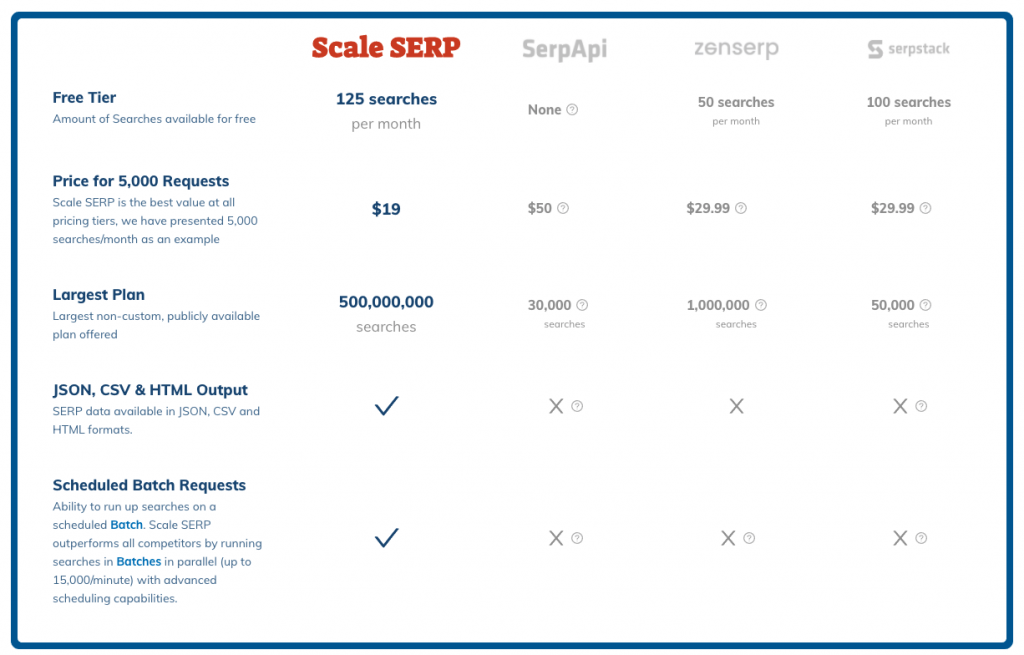
SERP scraping, while powerful, often encounters hurdles like CAPTCHAs, dynamic content, and API limitations. This section delves into advanced strategies to overcome these challenges and extract data more effectively and efficiently. Robust scraping requires adaptation to evolving website structures and security measures.
Advanced techniques are crucial for reliable data collection, particularly when dealing with websites that employ sophisticated anti-scraping mechanisms. These techniques enable scraping even when faced with dynamic content generation, CAPTCHAs, or rate-limiting issues.
Dealing with CAPTCHAs and Security Measures
CAPTCHAs are a common security measure to deter automated scraping. To bypass these, several approaches can be implemented. These include using CAPTCHA solvers, either through dedicated services or through custom-built solutions. Image recognition libraries and machine learning models can be trained to identify and solve CAPTCHAs. The selection of a method will depend on the complexity and frequency of the CAPTCHAs encountered.
Strategies for Scraping Dynamically Generated Content
Websites often employ JavaScript to generate content dynamically. Standard scraping techniques may fail to capture this content. Using tools like Selenium or Puppeteer, which can simulate a browser, is essential. These tools execute JavaScript code, rendering the page as a human user would see it, allowing for accurate data extraction. It’s vital to respect website terms of service and rate limits.
Using headless browsers provides a crucial step in accurately reflecting the data on the web pages.
Scraping APIs for Enhanced Data Retrieval
Many websites offer APIs for data access. Utilizing these APIs can often be a more structured and efficient approach compared to scraping web pages directly. APIs provide predefined endpoints and data formats, streamlining data retrieval. For example, Google Search Console API can be used to fetch structured data from Google search results, offering insights into website performance.
Utilizing APIs ensures compliance with the website’s terms of service and avoids potential issues with rate limits or anti-scraping mechanisms.
Using Proxies and Rotating IPs
To avoid being blocked by websites, using proxies and rotating IP addresses is a common practice. Proxies act as intermediaries between your scraping script and the target website. Rotating IPs simulate different user requests, making it harder for the target website to detect and block your scraping activity. Choosing a reliable proxy provider is crucial to avoid connection issues or unreliable proxies.
Using a proxy pool that provides rotating IPs helps to mitigate the risk of being detected as a bot.
Real-World Example of Advanced Scraping Techniques
Imagine scraping product reviews from an e-commerce site. The site uses AJAX requests to load reviews dynamically. A simple HTTP request would miss the reviews. Using Selenium with JavaScript execution, the scraper can load the page fully, rendering the dynamic content. The scraper then extracts the review data using Beautiful Soup.
This approach can be further enhanced by using a rotating proxy to handle potential rate-limiting issues. A robust implementation should include error handling to gracefully manage website changes and potential failures.
Learning how to perform SERP scraping in Python can be super useful for all sorts of projects. For example, imagine you’re trying to track public information about a recent federal employees firings lawsuit, like this one. You could use Python to gather data on search results related to that lawsuit. Then, you can process the data to analyze trends or gain insights into public perception.
It’s a cool technique for digging into trending topics!
Case Studies and Examples
SERP scraping, when done responsibly, can yield valuable insights into user search behavior, market trends, and competitive landscapes. This section presents practical case studies showcasing the application of SERP scraping techniques across various data types and use cases. We’ll examine ethical considerations and highlight best practices throughout.
Understanding the nuances of different SERP result types and the data they contain is crucial for effective scraping. This includes not only the typical web page results but also image, news, and video listings. Different scraping approaches are needed to effectively extract information from these diverse result types.
Scraping News Results, How to perform serp scraping in python
Extracting news articles from SERPs involves handling dynamic content and pagination. A crucial step is to identify the structure of the news snippets displayed. This often involves using libraries like Beautiful Soup to parse the HTML and extract relevant elements like article titles, publication dates, and links.
- Example: Scraping news articles related to a specific company from Google News. The scraper would identify the HTML elements containing news titles and links, then follow those links to fetch the full article content.
- Ethical Considerations: Respecting copyright and terms of service is paramount. It’s vital to obtain permission before scraping from sites that explicitly prohibit it. Excessive scraping can overwhelm the target website, potentially leading to server overload.
Scraping Image Results
Image results often have different display structures compared to regular web page results. The scraper needs to identify the image URLs, associated captions, and alt text.
- Example: A scraper could collect images of a specific product from a search query, extracting the image URLs, alt text, and potential product information associated with the images.
- Ethical Considerations: Ensure proper attribution of images and respect the copyright of the image owners. Don’t scrape images from sites with explicit “no scraping” policies.
Scraping Video Results
Scraping video results is often more complex due to the embedded nature of the video content within the SERP.
- Example: Collecting video results for a specific topic from YouTube, extracting the video titles, descriptions, and links to embed the videos in a report.
- Ethical Considerations: Respect the copyright and terms of service of video platforms like YouTube. Avoid scraping excessive amounts of video data to prevent overloading their servers.
Scraping Structured Data from SERPs
Many SERPs display structured data in tables, such as business information, product details, or movie reviews.
- Example: Scraping local business listings to create a database of local restaurants or shops, including their addresses, phone numbers, and customer reviews.
- Ethical Considerations: Maintain data accuracy and avoid misrepresenting the information scraped. Always check the website’s robots.txt file to understand their scraping policies.
Data Types and Scraping
This section focuses on scraping various data types from SERPs. For instance, scraping company profiles, product specifications, or price comparisons.
- Example: Scraping product listings to compare prices from different retailers, extracting data like product name, price, and retailer.
- Ethical Considerations: Respect the data privacy policies of the scraped websites. Always verify the legitimacy of the data before using it for any commercial purpose.
Tools and Resources for SERP Scraping
SERP scraping, while powerful, requires effective tools and resources to navigate the complexities of web data extraction. This section explores valuable aids, from dedicated libraries to helpful online communities, to ensure smooth and efficient scraping processes. Proper utilization of these resources can significantly streamline your project and prevent common pitfalls.
Effective SERP scraping hinges on leveraging readily available resources and tools. This includes understanding the intricacies of web scraping libraries, accessing relevant documentation, and utilizing online communities for support and collaboration. These resources will be critical in building robust and reliable scraping solutions.
Python Libraries for SERP Scraping
Python boasts a rich ecosystem of libraries designed for web scraping. Libraries like `requests` and `Beautiful Soup` are fundamental for fetching and parsing web pages. Beyond these, dedicated scraping frameworks like `Scrapy` offer robust solutions for handling complex tasks and ensuring scalability. These tools allow for efficient data extraction and management.
- `requests`: This library excels at making HTTP requests to fetch web pages. Its simplicity and ease of use make it a cornerstone for any scraping project.
- `Beautiful Soup`: `Beautiful Soup` is a powerful HTML/XML parser. It allows you to navigate and extract data from complex web structures, making it indispensable for data extraction.
- `Scrapy`: `Scrapy` is a high-level web scraping framework designed for efficient and scalable scraping. Its architecture allows for handling large volumes of data and complex websites, making it suitable for advanced projects.
- `Selenium`: This library is particularly useful for scraping websites that rely on JavaScript for rendering content. It allows you to interact with the browser and execute JavaScript, providing access to dynamically loaded data.
Documentation and Tutorials
Thorough documentation and comprehensive tutorials are vital for effective SERP scraping. These resources provide clear explanations, examples, and best practices for leveraging libraries and frameworks. They serve as valuable guides, ensuring you can effectively navigate the complexities of web data extraction.
- Official Library Documentation: Each Python library mentioned above has detailed documentation on their websites. These resources offer comprehensive explanations, examples, and code snippets.
- Online Tutorials and Guides: Numerous online tutorials and guides provide step-by-step instructions and practical examples for SERP scraping. These resources cater to varying skill levels, offering a spectrum of approaches to data extraction.
- Stack Overflow and Similar Communities: These online communities are valuable resources for troubleshooting and finding solutions to common issues. They offer a platform to connect with other users facing similar challenges and share knowledge.
Online Communities and Forums
Online communities and forums offer a crucial support system for SERP scrapers. They provide a platform for collaboration, knowledge sharing, and problem-solving. These resources allow for connecting with others who have experience in this field.
- Stack Overflow: A comprehensive question-and-answer site, where users can find answers to a wide range of web scraping questions, including those related to SERP scraping.
- Reddit Forums (r/webdev, r/programming): Reddit forums can provide insights and solutions related to web scraping techniques and tools. They can be valuable resources for community discussions and sharing experiences.
- Specific SERP Scraping Forums (if available): Dedicated forums dedicated to SERP scraping can provide specialized knowledge and insights from experienced users.
Tools for Testing and Validation
Tools for testing and validating the accuracy of your SERP scraping are crucial. These tools ensure that your scraper is functioning as expected and extracting the correct data. Robust testing procedures are essential for reliable results.
- Web Developer Tools (Browser Developer Tools): Built into most modern browsers, developer tools provide access to the underlying HTML and JavaScript code, allowing you to inspect the structure of web pages and identify data elements for extraction.
Final Summary
In conclusion, scraping SERPs with Python offers a valuable approach to data collection, but it’s crucial to respect website terms of service and robots.txt guidelines. This guide has provided a detailed roadmap for performing SERP scraping ethically and effectively. Remember to prioritize responsible scraping practices and always consider the ethical implications of your actions. By following the steps Artikeld here, you’ll be well-equipped to extract valuable insights from search engine results.