Ways to Fix Error 429 Too Many Requests
Ways to fix error 429 too many requests is a crucial topic for anyone dealing with APIs. This comprehensive guide dives into the intricacies of this common error, exploring various solutions from understanding the root causes to implementing sophisticated rate limiting strategies. We’ll cover everything from client-side handling to server-side mitigation, and even delve into caching and alternative approaches.
Error 429, “Too Many Requests,” often arises when applications send requests to an API at a rate exceeding its acceptable limit. Understanding the underlying causes and implementing effective solutions is key to preventing this error and ensuring smooth application functionality.
Understanding the Error 429
The dreaded “429 Too Many Requests” error is a common frustration for anyone interacting with online APIs or web services. It signals that your application is exceeding the server’s request rate limit, essentially being told “slow down.” This error is crucial to understand, as it impacts performance, data retrieval, and overall application functionality.The server imposes rate limits to manage its resources effectively and prevent overload.
Overwhelming the server with too many requests can lead to performance issues, data corruption, and even service outages. Understanding the underlying causes and strategies to overcome this error is essential for building robust and efficient applications.
Causes of Error 429
Excessive requests from a single client or a surge in requests from multiple clients are common causes. A poorly designed application that sends requests too frequently can trigger this error. Malicious actors may also use automated tools to generate a high volume of requests, potentially targeting a specific API or service. Inaccurate or inefficient algorithms within the application’s code can inadvertently cause the application to exceed the rate limit.
Temporary vs. Permanent Rate Limits
Rate limits can be temporary, meaning they are in place for a specific duration, perhaps a few minutes or hours. They are often used to handle peak usage periods, ensuring that the service remains responsive. Permanent rate limits, however, are designed to control the maximum number of requests allowed over a longer period or indefinitely. This is crucial for resource management and to prevent abuse.
Temporary rate limits often reset after a set time, allowing the application to resume operations. Permanent limits require the application to adjust its request strategy to adhere to the service’s constraints.
HTTP Status Codes
Understanding HTTP status codes is vital for troubleshooting. They provide crucial information about the outcome of a request. A comprehensive overview is presented in the table below.
HTTP Status Code | Description |
---|---|
200 | OK |
400 | Bad Request |
401 | Unauthorized |
403 | Forbidden |
404 | Not Found |
429 | Too Many Requests |
500 | Internal Server Error |
503 | Service Unavailable |
The table above illustrates various HTTP status codes, including the crucial 429 “Too Many Requests” error. Each code conveys specific information about the request’s outcome.
Rate Limiting Strategies
Rate limiting is a crucial aspect of building robust APIs and web applications. It prevents overwhelming servers with excessive requests, ensuring a smooth user experience and protecting resources from abuse. Understanding and implementing effective rate limiting strategies is essential for any application handling a significant volume of requests.Implementing rate limiting involves carefully controlling the frequency of requests from a given source, ensuring that the system doesn’t get overloaded.
This often involves techniques like delaying requests, using queuing systems, and employing backoff mechanisms to avoid the dreaded “429 Too Many Requests” error. A well-designed rate-limiting strategy ensures that the application remains responsive and avoids disruptions for legitimate users.
Delaying Requests
Delayed requests are a fundamental approach to rate limiting. When a request exceeds the defined rate limit, the application delays the subsequent request for a specified duration. This simple strategy can effectively reduce the load on the server, allowing it to process requests at a manageable pace.This method is straightforward to implement, but it can lead to increased latency for users.
Dealing with a “429 Too Many Requests” error can be a real pain, especially when you’re trying to access something fast, like live game updates. One common fix is to use a delay between requests. Another is to look at using a different API key, or even explore whether rate limiting is in effect. For example, if you’re looking at the CIF NorCal Championship soccer game between Mountain View Sacred Heart Prep and Piedmont, this exciting matchup might be experiencing high traffic, which could lead to rate-limiting issues.
Ultimately, understanding the source of the error and employing the right strategy to avoid the 429 is key.
The duration of the delay must be carefully chosen to balance the need for rate limiting with the user experience. For example, delaying requests by a few seconds can significantly reduce the load on the server while only slightly impacting the user’s experience, if the application is designed to handle this latency.
Using Queuing Systems
Queuing systems provide a more sophisticated approach to rate limiting. Instead of delaying individual requests, a queuing system buffers incoming requests that exceed the rate limit. The system then processes these requests in an orderly fashion, ensuring that the server is not overwhelmed.Using a queuing system can lead to a more predictable response time for users, especially in high-traffic scenarios.
However, the implementation complexity is often higher compared to simply delaying requests. The choice between delaying requests and using a queue often depends on the specific application requirements and the anticipated volume of traffic. Choosing the correct queuing system depends on factors such as the type of application, the volume of traffic, and the desired level of performance.
Exponential Backoff
Exponential backoff is a strategy for handling temporary failures, often used in conjunction with rate limiting. When a request fails due to rate limiting, the application delays subsequent requests by an exponentially increasing amount of time.This strategy helps to prevent further issues by reducing the frequency of requests during periods of high demand. For instance, if a request fails, the next attempt might be delayed by 1 second, then 2 seconds, then 4 seconds, and so on.
This ensures that the application doesn’t flood the server with requests and helps to recover from temporary overload conditions. The specific exponential growth factor should be carefully selected to balance the need for recovery with the impact on user experience.
Implementing Request Throttling (Examples)
Implementing request throttling in different programming languages is relatively straightforward. Here are examples using Python and JavaScript:
Python
“`pythonimport timedef rate_limited_function(func): def wrapper(*args,
*kwargs)
# Check if the rate limit has been exceeded if rate_limit_exceeded(): sleep_time = calculate_sleep_time() time.sleep(sleep_time) return func(*args, – *kwargs) return wrapper“`
JavaScript
“`javascriptfunction rateLimited(func, timeInterval) let lastCalled = 0; return function (…args) const now = Date.now(); if (now – lastCalled < timeInterval) setTimeout(() => func(…args), timeInterval – (now – lastCalled)); else lastCalled = now; func(…args); ;“`
Rate Limiting Libraries/Tools Comparison
| Library/Tool | Features | Pros | Cons ||—|—|—|—|| `ratelimit-axios` (JavaScript) | Simple rate limiting for Axios requests. | Easy to use, integrates well with Axios. | Limited customization options. || `python-limiter` (Python) | Offers various rate limiting strategies. | Flexible, supports different algorithms.
| Potentially more complex to set up. || `requests-rate-limit` (Python) | Designed specifically for the `requests` library. | Seamless integration, handles request delays gracefully. | Might not be suitable for all use cases. || …
Dealing with error 429 “Too Many Requests” can be a real headache, but thankfully, there are some easy fixes. One common culprit is making too many requests to a server too quickly. Slowing down your requests, using a rate-limiting library, or employing caching strategies can help. Speaking of headaches, have you checked out the spooky tale of love in Oakland?
It’s a gripping read, like something straight out of a horror movie. freaky tales oakland love song is a fascinating look into a darker side of the city, and it might even help inspire a more creative approach to handling those 429 errors. Ultimately, remember to be patient and methodical when you’re trying to overcome these API limitations.
(Other Libraries) … | … | … | … |
API Documentation and Rate Limits
Finding out how many API requests you can make within a specific timeframe is crucial for preventing the dreaded 429 error. Understanding rate limits directly impacts your application’s performance and stability. This section delves into how to locate and interpret these limits within API documentation, and how to design your own APIs with robust rate limiting.API documentation is your primary resource for uncovering rate limits.
It’s the single source of truth for how frequently you can access an API endpoint. These details often reside in the documentation’s specific sections dedicated to usage policies, performance guidelines, or frequently asked questions.
Locating API Rate Limits
API rate limits are typically documented within the API’s usage policies. This information often describes the allowed number of requests within a certain timeframe, such as requests per minute, hour, or day. Sometimes, the documentation may use terms like “quota,” “allowance,” or “limit.” Look for sections clearly outlining these parameters.
Interpreting Rate Limit Information
Understanding the format of rate limit information is essential. Rate limits are often expressed as requests per unit of time. For example, “100 requests per minute” means you can send up to 100 requests to the API every 60 seconds. Carefully examine the units (minute, hour, day) to avoid exceeding the limits.
Examples of Rate Limit Headers
Rate limits are often communicated through HTTP headers in API responses. These headers provide crucial information about the remaining requests within a given time frame.
- X-RateLimit-Limit: Specifies the total number of requests allowed within a given timeframe (e.g., 100 requests per minute).
- X-RateLimit-Remaining: Indicates the number of requests remaining before the limit is reached.
- X-RateLimit-Reset: This value indicates when the rate limit will reset, usually in seconds from the current time.
- X-RateLimit-Retry-After: This header tells how long you need to wait to make another request if you’ve exceeded the rate limit. It’s critical for implementing appropriate retry logic.
These headers are fundamental to properly managing your API requests and avoiding the 429 error.
Implementing Rate Limiting in Your API Design
Implementing rate limiting in your own API design is a crucial defensive measure. It protects your API from abuse and ensures fair access for all users. Consider these factors:
- API Keys: Associate rate limits with specific API keys, enabling granular control over requests per key. This approach allows you to differentiate between various users and their request volumes.
- IP Addresses: Rate limit requests based on IP addresses, particularly useful for preventing denial-of-service attacks. A high volume of requests from a single IP address could trigger a rate limit.
- Request Frequency: Track the frequency of requests from individual users or applications to prevent abuse.
Rate Limiting Header Fields
A table summarizing common rate limiting header fields and their meanings:
Header Field | Meaning |
---|---|
X-RateLimit-Limit | Total requests allowed within a specific time frame. |
X-RateLimit-Remaining | Number of requests remaining before the limit is reached. |
X-RateLimit-Reset | Timestamp when the rate limit resets (usually in seconds). |
X-RateLimit-Retry-After | Time to wait before retrying the request if the limit has been exceeded. |
Debugging and Troubleshooting
Pinpointing the source of a 429 error, “Too Many Requests,” requires a systematic approach. Often, the problem lies in how frequently your application is interacting with the API. Understanding the API’s rate limits and adjusting your requests accordingly is crucial. This section delves into practical debugging steps, header inspection, monitoring tools, and testing techniques to effectively resolve 429 errors.Identifying the root cause involves investigating your application’s interaction with the API, examining request patterns, and checking the API documentation for rate limits.
Troubleshooting 429 errors is often about aligning your application’s request frequency with the API’s acceptable rate.
Common Debugging Steps for 429 Errors
Effective debugging starts with a clear understanding of the potential causes. Below are key steps to follow when encountering a 429 error:
- Review your API requests. Analyze the frequency and timing of requests. Are they being sent too quickly? Are they grouped in bursts?
- Check your application’s code for any loops or processes that might be sending requests excessively.
- Inspect your code for asynchronous tasks that might be launching multiple requests concurrently without proper synchronization.
- Confirm if you’re using any libraries or frameworks that could be contributing to excessive requests, and ensure you are using proper request queuing or throttling mechanisms if needed.
Inspecting HTTP Headers for Rate Limit Information, Ways to fix error 429 too many requests
The HTTP response headers often contain crucial information about the rate limit. Examining these headers can quickly reveal the current status of your requests and the remaining allowance.
- Look for headers like `X-RateLimit-Limit`, `X-RateLimit-Remaining`, and `X-RateLimit-Reset`. These headers provide insights into the rate limit’s maximum requests, the requests remaining, and the time until the reset.
- Analyzing these values helps you understand how many more requests you can send before hitting the limit.
- If the `X-RateLimit-Remaining` value is close to zero, your application is approaching the rate limit and needs adjustment.
Using Tools to Monitor API Usage
Monitoring tools and network analyzers offer valuable insights into your application’s API interactions. These tools help identify patterns and potential issues.
- Utilize network analyzers (like Wireshark) to capture and examine network traffic. These tools can reveal the timing and frequency of your API calls.
- Employ API monitoring tools to track requests, responses, and error rates. Such tools provide detailed reports on your API usage and identify trends that might indicate excessive requests.
- Integrate logging mechanisms in your application to record request details, including timestamps and response codes. This data helps you understand request patterns and potential bottlenecks.
Testing Different Request Frequencies
Testing different request frequencies is crucial for pinpointing the optimal rate for your application. This method helps avoid rate limits and maintain smooth API interactions.
- Experiment with varying request intervals to find the maximum frequency that doesn’t trigger the 429 error. This helps establish the API’s tolerance for requests.
- Use tools or techniques to simulate realistic request patterns, such as using a load testing tool to create consistent, simulated traffic.
- Gradually increase the frequency of requests and observe when the 429 error occurs. This helps determine the API’s threshold for acceptable request volume.
Potential Issues and Solutions
This table summarizes common issues leading to 429 errors and corresponding solutions:
Issue | Solution |
---|---|
Rapid request bursts | Implement request throttling or queuing mechanisms to space out requests. |
Asynchronous tasks without synchronization | Use appropriate synchronization mechanisms to control the concurrent execution of asynchronous tasks. |
Issues with API libraries or frameworks | Update libraries or frameworks to the latest versions or explore alternative solutions if the problem persists. |
Incorrect request intervals | Adjust the intervals between requests to align with the API’s rate limit. |
Client-Side Handling: Ways To Fix Error 429 Too Many Requests
Handling rate-limiting errors on the client-side is crucial for maintaining application responsiveness and preventing service disruptions. A well-designed client-side approach ensures smooth user experience by gracefully managing requests that exceed the API’s allowed rate. Properly implemented retry mechanisms and delay strategies prevent excessive hammering of the API, preserving its availability for all users.Effective client-side handling of error 429 involves proactive measures to avoid overwhelming the server.
By strategically pausing requests and adjusting retry attempts, applications can prevent their requests from triggering further rate-limiting issues. This proactive approach ensures continued operation even when encountering temporary limitations imposed by the API.
Retry Mechanisms for Rate-Limited Requests
Implementing robust retry mechanisms for rate-limited requests is vital for maintaining application functionality and user experience. These mechanisms allow applications to handle temporary throttling issues without impacting the overall flow of operations. By intelligently retrying requests after a predefined delay, applications can avoid overloading the API and ensure data retrieval or other actions are completed successfully.
- Exponential Backoff: Exponential backoff is a common strategy where the retry delay increases exponentially after each failed attempt. This approach prevents the client from flooding the server with requests and gives the server time to recover. For example, if the initial delay is 1 second, the next retry could be 2 seconds, then 4 seconds, and so on.
This ensures that the requests are spaced out over time, allowing the server to manage the load more effectively.
- Jitter: Adding a random delay (jitter) to the exponential backoff strategy prevents clients from making requests simultaneously. This randomization helps to distribute requests over time and further reduces the impact on the server. Adding jitter avoids a predictable pattern in requests, which can exacerbate the problem.
- Retry Limits: Setting a maximum number of retries is essential to prevent infinite loops. This limit ensures that the client doesn’t keep retrying indefinitely if the server is persistently unavailable. This helps avoid indefinite blocking and keeps the application responsive.
Managing Request Delays
Appropriate management of request delays is critical for handling rate-limited requests gracefully. This involves implementing strategies that prevent overwhelming the server while ensuring timely responses to the user. Implementing delays prevents the client from flooding the server with requests, preserving its availability.
- Fixed Delays: Using a fixed delay between requests can be sufficient for some APIs. However, this method is not ideal for APIs with dynamic rate limits. A constant delay may not be appropriate for APIs with varying rates or unpredictable responses.
- Adaptive Delays: Employing adaptive delays based on the server’s response is crucial for efficient rate-limiting handling. This approach allows the client to adjust the delay based on the API’s response. This ensures the client responds dynamically to server feedback.
- Dynamic Delay Strategies: Utilizing dynamic delay strategies based on the rate limit is essential. This strategy allows the client to adjust the delay dynamically to align with the server’s current capacity. This approach ensures the client adjusts the delay based on the server’s current rate limit.
Implementing Exponential Backoff in Client-Side Code
Implementing exponential backoff in client-side code involves calculating an increasing delay between retries. This approach is suitable for APIs with rate limits to prevent overwhelming the server. The delay increases exponentially to give the server time to recover.
Example (Conceptual Python):“`pythonimport timeimport randomdef exponential_backoff(initial_delay, max_delay): delay = initial_delay while True: # … make request … if success: return delay = min(delay
2, max_delay)
delay += random.uniform(0, delay / 2) # Add jitter time.sleep(delay)“`
Using Libraries for Client-Side Rate Limiting
Leveraging libraries for client-side rate limiting simplifies the implementation process. These libraries handle the complexities of retry mechanisms and delay strategies, allowing developers to focus on application logic. These libraries streamline the process of rate limiting by providing pre-built solutions.
- `requests` (Python): The `requests` library, while primarily for HTTP requests, can be combined with other libraries or custom functions to implement rate limiting logic. This approach offers flexibility and control over the rate limiting process.
- Dedicated Rate Limiting Libraries: Libraries specifically designed for rate limiting provide a more structured and efficient way to handle requests, including backoff strategies. These libraries provide comprehensive support for various rate-limiting scenarios.
Server-Side Mitigation
Protecting your API from abuse is crucial. Server-side rate limiting is a vital defense mechanism against overwhelming requests, preventing denial-of-service attacks and ensuring fair resource allocation. This approach directly controls the rate at which clients can access your API endpoints, safeguarding against potential harm and maintaining a responsive system.Implementing rate limits on the server side allows for granular control over request frequency, preventing abuse and optimizing performance.
This strategy often involves examining request patterns and adjusting limits based on client behavior, IP address, or specific API endpoints. This proactive approach to managing resource usage is key to maintaining API health and reliability.
Rate Limiting Strategies
Implementing effective rate limiting involves careful consideration of the desired constraints. A simple approach involves tracking requests per second or minute for a specific client or IP address. More sophisticated strategies utilize sliding windows or exponentially decaying counters to accommodate varying request patterns.
- Request Counting: This basic method maintains a count of requests from a client or IP address within a specific timeframe (e.g., 1 minute). If the count exceeds the predefined limit, further requests are rejected. This approach is straightforward but can be easily overwhelmed by bursts of requests.
- Sliding Window: A sliding window approach considers requests within a moving time window. Instead of tracking all requests in a fixed interval, the window slides over time, focusing on recent activity. This method provides a more responsive and adaptive rate limit compared to a fixed-interval counter.
- Token Bucket: This strategy maintains a bucket that fills with tokens at a constant rate. Clients can only access the API if tokens are available. If the bucket is empty, requests are queued or rejected. This method is particularly useful for handling bursty traffic, as it allows for temporary bursts of requests without immediate rejection.
- Leaky Bucket: A leaky bucket allows requests to enter the bucket at a constant rate. Tokens are removed from the bucket at a constant rate, mimicking a leaky bucket. If the bucket fills, new requests are rejected. This approach can handle a steady stream of requests.
Controlling Request Frequency
Implementing controls based on client or IP address allows for targeted restrictions. A common approach is to maintain separate rate limit counters for different clients or IP ranges, enabling tailored control over resource usage.
- Client-Specific Limits: Different clients might have different request rates based on their roles or needs. This allows for customization, ensuring that clients with higher demands are not penalized.
- IP Address-Based Limits: Restricting requests from specific IP addresses can help identify and mitigate malicious activity or abusive patterns. This is particularly important for protecting against denial-of-service attacks.
Token Buckets and Leaky Buckets
These techniques are vital for managing bursty traffic patterns. Understanding their differences is key to implementing effective rate limits.
Token buckets maintain a bucket of tokens that fill at a constant rate. Clients can consume tokens to access the API. A leaky bucket, on the other hand, allows requests to enter the bucket at a constant rate but removes tokens at a fixed rate, providing a steady flow of access.
Server-Side Libraries and Tools
Several libraries and tools simplify rate limiting implementation in various programming languages. These tools often provide functionalities like configuration management, request logging, and error handling.
- Python Libraries (e.g., `ratelimit`): These libraries offer pre-built rate limiting mechanisms, reducing development time and ensuring consistency.
- Java Libraries (e.g., `Guava`): Java libraries provide efficient and versatile rate limiting functionalities, suitable for various applications.
- Redis for Rate Limiting: Redis, a popular in-memory data store, can be leveraged for efficient rate limiting, storing and retrieving rate limit data.
Server-Side Rate Limiting Configurations
A well-structured approach ensures that rate limits are tailored to specific needs. A table Artikels different configurations.
Configuration | Description | Use Case |
---|---|---|
Client-based limit (10 requests/minute) | Limits requests from each client to 10 per minute. | Protecting against abuse by a single user. |
IP-based limit (50 requests/minute) | Limits requests from each IP address to 50 per minute. | Mitigating denial-of-service attacks. |
Endpoint-specific limit (200 requests/second) | Limits requests to a specific endpoint to 200 per second. | Protecting the server from overload on a specific resource. |
Caching and Prefetching
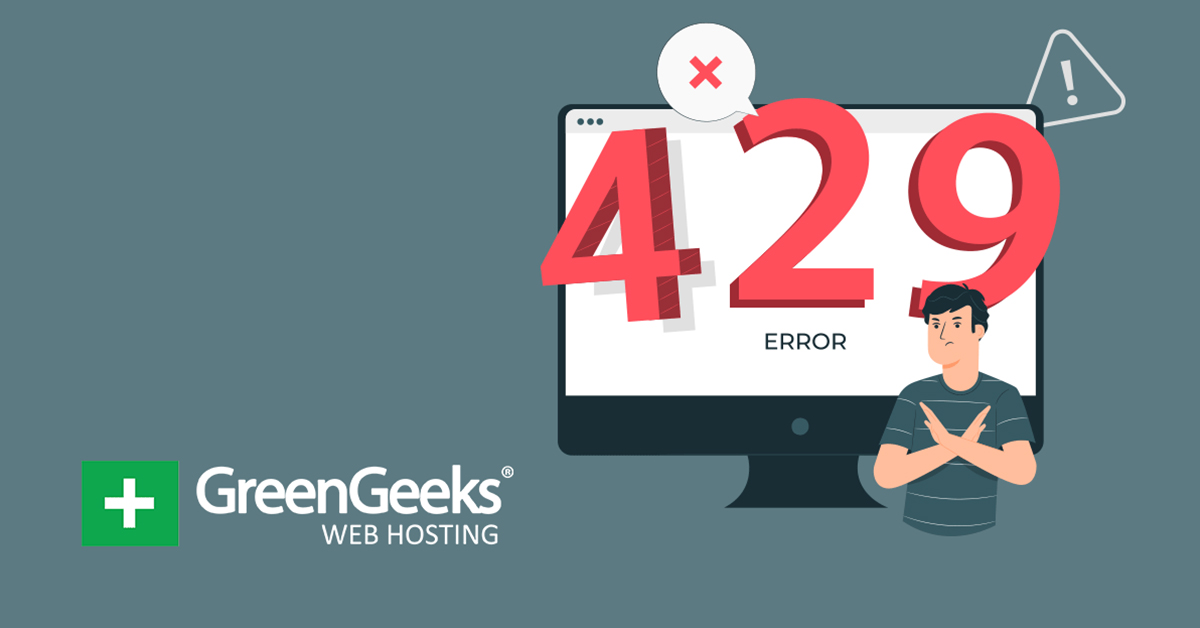
Caching and prefetching are crucial strategies for optimizing API performance and mitigating 429 errors. By storing frequently accessed data and anticipating future requests, these techniques significantly reduce the load on the API, leading to improved responsiveness and reliability. These strategies are especially important when dealing with large volumes of requests or fluctuating demand.Caching acts as a temporary storage location for data that is frequently accessed.
Dealing with that dreaded “429 Too Many Requests” error can be a real pain, but there are ways to fix it. Sometimes, it’s just a case of waiting a bit. For instance, if you’re dealing with a website experiencing a high volume of traffic, or maybe you’re unintentionally overloading a system, you might want to take a break before trying again.
Learning about user experience, like in the case of miss manners food allergies , can also teach us to be more mindful of the resources we consume. Ultimately, understanding rate limits and adjusting your approach can resolve those pesky errors, allowing you to keep your online adventures flowing smoothly.
This reduces the need to retrieve the data from the original source, significantly accelerating response times. Prefetching, on the other hand, proactively fetches data that is likely to be requested in the near future, further minimizing latency. Together, these techniques create a more efficient and resilient API infrastructure.
Caching Strategies for API Requests
Caching frequently accessed data dramatically reduces the number of requests to the backend API. This reduces the load on the API server, preventing 429 errors. Appropriate caching strategies can improve the application’s performance significantly.
- Data-Based Caching: This approach caches data based on specific criteria like user IDs, product IDs, or timestamps. For example, a user’s profile information might be cached for a specific duration after the initial retrieval. This minimizes repeated calls to the API to retrieve the same data.
- Request-Based Caching: Caching entire responses to specific API requests. This approach is ideal when the same request patterns occur frequently. For instance, retrieving the list of available products is a good candidate for this type of caching.
- Expiration-Based Caching: This method involves setting a time limit for the cached data’s validity. After the expiration time, the data is considered stale and needs to be refreshed from the source. This approach balances performance with data freshness. A common use case is for information that is likely to change frequently, such as real-time stock quotes or prices.
Implementing Caching Mechanisms
Various caching mechanisms are available for implementing caching strategies. Choosing the right mechanism depends on factors such as performance requirements, data volume, and desired caching duration.
- Redis: A popular open-source, in-memory data structure store. Redis excels at handling high-volume data and offers various data structures for efficient caching. Its speed and flexibility make it suitable for demanding applications. Redis is often used in combination with other caching strategies.
- Memcached: Another widely used, high-performance caching system. Memcached is optimized for caching simple data types like strings and numbers. It’s particularly efficient for caching static content or frequently accessed data. Memcached is known for its speed and scalability.
- In-Memory Caching: Some programming languages offer built-in caching mechanisms. This approach is often simpler to implement and manage, but may have limited scalability compared to dedicated caching systems like Redis or Memcached.
Prefetching Strategies
Anticipating future requests is crucial for mitigating 429 errors. Prefetching strategies can be implemented by proactively retrieving data that is likely to be requested in the near future.
- Predictive Prefetching: This approach relies on analyzing historical request patterns to predict future requests. For example, if a user frequently requests data for specific product categories, the system can prefetch the data for those categories before the user actually requests them.
- User-Based Prefetching: Prefetching data based on user activity and preferences. If a user frequently visits specific pages or accesses particular resources, the system can proactively load related content.
Comparison of Caching Strategies
The choice of caching strategy depends on the specific needs of the application.
Strategy | Description | Advantages | Disadvantages |
---|---|---|---|
Data-Based | Caches data based on specific criteria. | Efficient for retrieving data associated with specific entities. | May require complex logic to determine appropriate caching keys. |
Request-Based | Caches entire responses to specific API requests. | Simple implementation for frequently accessed data. | Can lead to large cache sizes if not managed effectively. |
Expiration-Based | Caches data with a defined expiration time. | Ensures data freshness. | Requires careful consideration of expiration times to avoid stale data. |
Alternative Approaches
Rate limiting, while crucial for API health, can sometimes hinder performance. When dealing with high-volume requests, or when the nature of the requests necessitates bypassing rate limits, alternative solutions become vital. These approaches often involve queuing systems to manage the influx of requests and message brokers for asynchronous processing.
Queuing Systems for Handling Bursts
Queuing systems act as a buffer between the incoming requests and the backend processing. They store requests that exceed the rate limit, ensuring that they are processed eventually. This approach is particularly useful for handling bursts of requests, such as during peak hours or promotional campaigns. Choosing the right queuing strategy is critical to maintaining application responsiveness and performance.
Benefits and Drawbacks of Different Queuing Strategies
Different queuing strategies offer various trade-offs in terms of performance, reliability, and complexity. FIFO (First-In, First-Out) queues are simple and straightforward, but might not be optimal for prioritizing requests. Priority queues allow for assigning different priorities to requests, enabling critical tasks to be processed before less urgent ones. LIFO (Last-In, First-Out) queues, often used for undo operations, are less suitable for handling a high volume of diverse requests.
Each strategy has its strengths and weaknesses, and the best choice depends on the specific needs of the application.
Using Message Brokers for Asynchronous Request Processing
Message brokers are intermediary systems that facilitate communication between different components of an application, often used for asynchronous request processing. This approach decouples the request generation from the actual processing, allowing the application to handle a high volume of requests without being bogged down by immediate processing. Message brokers provide a reliable mechanism for handling requests that exceed rate limits, allowing the application to remain responsive during peak loads.
Comparison of Queuing Systems
Queuing System | Features | Pros | Cons |
---|---|---|---|
Redis | In-memory data structure store, supports various data types including lists (for queues), offers high performance, and scalability. | High speed, simple implementation, flexible data structures | Requires server maintenance, potential for data loss if not configured correctly. |
RabbitMQ | Message broker, open-source, robust, supports various message queuing patterns, provides reliable delivery guarantees. | Reliable message delivery, high scalability, flexible message routing. | More complex setup compared to Redis, requires more expertise in message broker configuration. |
Kafka | Distributed streaming platform, designed for high-throughput data pipelines, ideal for handling massive volumes of data. | High throughput, fault tolerance, scalability | Complex configuration, requires expertise in distributed systems. |
Amazon SQS | Fully managed message queue service from AWS, offers scalability, reliability, and ease of use. | Ease of use, managed service, scalable | Requires AWS account and costs associated with usage. |
Closure
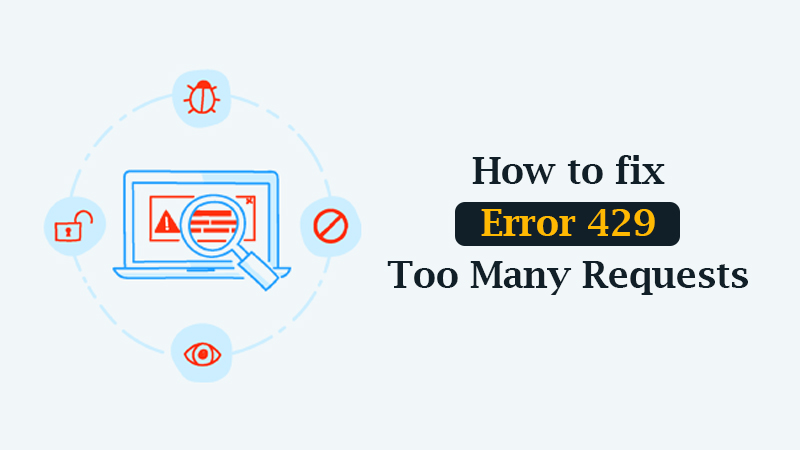
In conclusion, fixing error 429 requires a multi-faceted approach. By understanding rate limits, implementing appropriate strategies on both client and server sides, and utilizing caching techniques, you can effectively prevent this error and optimize your application’s performance. Remember that the ideal solution will depend on your specific application and API, so tailoring your approach is essential.