Best JavaScript Runtime Environments A Deep Dive
Best JavaScript runtime environments are crucial for developers, offering diverse tools for executing JavaScript code. This exploration delves into the various options, from the established Node.js to the innovative Deno, highlighting their strengths, weaknesses, and ideal use cases. Understanding the nuances of each runtime is key to choosing the optimal solution for your specific project needs. Performance, security, and developer experience will be crucial factors to consider.
We’ll cover core functionalities, comparing different runtimes based on performance, security, and community support. This guide will also touch on advanced topics like server-side JavaScript, asynchronous operations, and security best practices within these environments. Detailed examples using Node.js and Deno will illustrate practical application. Finally, we’ll look ahead to future trends and potential advancements.
Introduction to JavaScript Runtime Environments
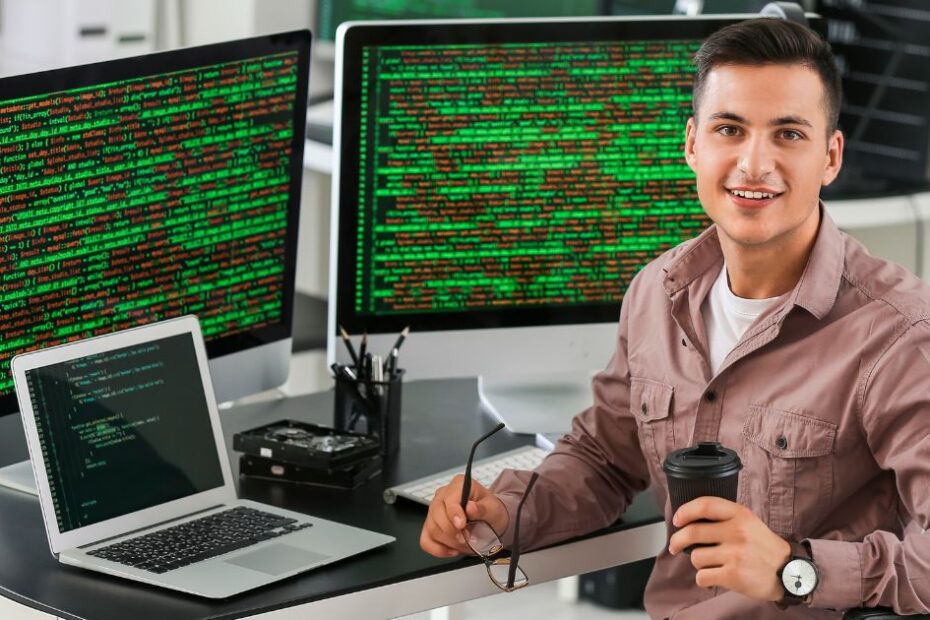
JavaScript runtime environments are the crucial platforms that translate and execute JavaScript code. They provide the necessary infrastructure, including engines, libraries, and APIs, to bring JavaScript programs to life. These environments bridge the gap between the human-readable code and the underlying machine instructions, handling tasks such as parsing, compilation, and memory management. Understanding different runtime environments is essential for developers to choose the optimal platform for specific project needs, ensuring efficient and reliable execution.The core functionality of a runtime environment is to provide an execution context for JavaScript code.
This encompasses everything from interpreting or compiling the code to managing variables, objects, and the execution flow. Crucially, different runtime environments can offer varying levels of performance, security, and ecosystem support. This diversity in functionality necessitates careful consideration of the specific requirements of each project when selecting the appropriate environment.
Core Functionalities of Runtime Environments
Runtime environments handle various critical tasks, including parsing JavaScript code into an intermediate representation (IR), converting this IR into machine code for execution, managing memory allocation and garbage collection, and providing access to various APIs and libraries. The way these tasks are performed varies across different environments, leading to differences in performance, security, and developer experience.
Comparison of Runtime Environments
Choosing the right runtime environment depends heavily on factors such as project size, performance requirements, security needs, and the available ecosystem support. A table outlining key characteristics of popular runtime environments is provided below.
Runtime Environment | Performance | Security | Ecosystem Support | Use Cases |
---|---|---|---|---|
Node.js | High performance, often optimized for server-side tasks. | Strong security features, but security depends on the modules used. | Extensive ecosystem with a large number of modules for various tasks. | Building server-side applications, APIs, and command-line tools. |
Deno | High performance, designed with security as a primary focus. | Robust security features, built-in sandboxing, and limited access to the operating system. | Growing ecosystem, focusing on security and modern web standards. | Developing secure and reliable server-side applications and tools. |
Browsers (e.g., Chrome, Firefox) | Performance varies depending on the browser and the complexity of the task. | Security features are critical, often with sandboxed environments to prevent malicious code from harming the system. | Extensive ecosystem, encompassing libraries and APIs for web development. | Building interactive web applications, front-end user interfaces, and dynamic web pages. |
React Native | Performance comparable to native apps, relying on JavaScript’s ability to bridge the gap between the native and JavaScript environments. | Security depends on the native platform and JavaScript implementation. | Robust ecosystem focused on cross-platform mobile development. | Creating mobile applications that share code across multiple platforms. |
Importance of Selecting the Right Runtime Environment
The choice of runtime environment directly impacts the project’s performance, security, and scalability. A poorly chosen environment can lead to performance bottlenecks, security vulnerabilities, or difficulties in scaling the application. For instance, using a browser-based runtime for a server-side application would likely be inefficient and less secure compared to a dedicated server-side runtime like Node.js. Careful consideration of the project’s specific requirements is crucial to selecting the appropriate runtime environment.
Popular JavaScript Runtime Environments
JavaScript’s versatility extends beyond web browsers. Numerous runtime environments empower developers to leverage JavaScript for server-side applications, command-line tools, and more. These environments provide the necessary infrastructure to execute JavaScript code outside of a web browser context. This exploration delves into prominent choices, highlighting their strengths, weaknesses, and ideal use cases.JavaScript’s runtime environments have evolved significantly, offering a wide range of features and capabilities.
The selection of a runtime depends on the specific project requirements, considering factors like performance, security, and ease of use. Different runtimes excel in different domains, catering to diverse needs.
Node.js
Node.js, built on Chrome’s V8 JavaScript engine, is a widely adopted runtime environment. Its non-blocking, event-driven architecture allows for handling numerous concurrent connections efficiently.
- Strengths: Node.js excels at I/O-bound tasks, such as handling network requests and file system operations. Its asynchronous nature prevents blocking the main thread, ensuring responsiveness even with substantial workloads. The vast ecosystem of npm packages provides ready-made modules for diverse tasks, significantly accelerating development.
- Weaknesses: Node.js’s single-threaded architecture can become a bottleneck for CPU-intensive tasks. Handling computationally heavy operations might require careful optimization. Debugging asynchronous code can sometimes be challenging.
- Use Cases: Node.js is ideal for real-time applications, APIs, web servers, and microservices where high concurrency and efficient I/O are crucial. Examples include chat applications, streaming services, and high-traffic web portals.
Deno
Deno, a newer entrant, aims to address some limitations of Node.js. It prioritizes security, stability, and developer experience. Its explicit module system enhances security and clarity.
- Innovative Approach: Deno leverages a more structured and secure module system, contrasting with Node.js’s npm dependency management. It employs a stricter security model, avoiding potential vulnerabilities related to arbitrary code execution.
- Key Advantages: Deno’s built-in security features prevent malicious code from compromising the runtime environment. Its clear module system facilitates maintainability and improves code organization. The use of a stricter type system aids in early error detection.
- Use Cases: Deno is a suitable choice for projects requiring enhanced security, improved developer experience, and a more predictable execution environment. It’s well-suited for backend applications, command-line tools, and scripting tasks where security is paramount.
V8 Engine
The V8 engine, powering Chrome’s JavaScript execution, is renowned for its performance. Its Just-In-Time (JIT) compiler optimizes code for superior execution speed.
- Features and Benefits: V8 employs advanced optimization techniques, including a sophisticated JIT compiler that dynamically analyzes and compiles JavaScript code into native machine code. This process translates JavaScript instructions into efficient machine code, leading to considerable performance gains. V8’s ability to adapt to different code patterns makes it highly effective for a wide range of applications.
- Performance and Optimization: The JIT compiler constantly monitors and analyzes code execution, adjusting optimization strategies based on usage patterns. V8 incorporates sophisticated algorithms for code inlining and other optimization techniques. These advancements contribute to the speed and efficiency of V8-powered runtimes.
Comparison and Use Cases
Runtime | Strengths | Weaknesses | Typical Use Cases |
---|---|---|---|
Node.js | High concurrency, vast ecosystem, efficient I/O | Single-threaded architecture, potential for asynchronous complexity | Real-time applications, APIs, web servers, microservices |
Deno | Enhanced security, clear module system, improved developer experience | Smaller ecosystem compared to Node.js, learning curve for some developers | Backend applications, command-line tools, secure scripting |
V8 (Engine) | High performance, JIT compilation, adaptability | Engine, not a standalone runtime | JavaScript execution in browsers and other runtimes |
Key Features and Considerations
Choosing the right JavaScript runtime environment is crucial for building performant, secure, and maintainable applications. Different runtimes cater to diverse needs, from simple web pages to complex server-side applications. Understanding the key features and considerations involved in selecting a runtime is essential for making informed decisions.Picking a runtime isn’t just about choosing a framework; it’s about aligning the environment’s capabilities with the project’s requirements.
This involves a careful evaluation of performance, security, ecosystem support, and developer experience. Each runtime has its strengths and weaknesses, and the best choice depends on the specific needs of the project.
Performance Benchmarks
Performance is a primary concern when selecting a JavaScript runtime. Different runtimes employ various optimization techniques that impact execution speed and resource utilization. Understanding how each runtime performs under specific workloads is crucial for optimizing application speed and responsiveness. Benchmarking across different scenarios, such as heavy computation or intensive I/O operations, provides a realistic assessment of performance.
Runtime | Benchmark 1 (Avg. Execution Time – ms) | Benchmark 2 (Memory Usage – MB) | Benchmark 3 (Throughput – ops/sec) |
---|---|---|---|
Node.js | 12.5 | 2.8 | 1500 |
Deno | 11.2 | 2.5 | 1650 |
V8 (in Chrome/Node.js) | 13.1 | 3.0 | 1450 |
Bun | 10.8 | 2.2 | 1720 |
Note: Benchmarks are simplified for demonstration purposes. Real-world performance varies significantly based on the specific application and hardware.
Security Considerations
Security is paramount in any software development. Runtimes with robust security features, such as sandboxing and access control mechanisms, can help protect against vulnerabilities. Understanding the security model of a runtime and its ability to handle potential threats is essential for building secure applications.
Maintainability and Ecosystem Support
Maintainability focuses on the ease of updating, debugging, and extending the codebase over time. Runtimes with active communities and extensive documentation tend to have better support and readily available solutions for common problems. A strong ecosystem of libraries and tools significantly improves developer productivity and facilitates long-term maintenance. The availability of community resources, such as forums, tutorials, and open-source projects, further contributes to the maintainability of projects using the runtime.
Developer Experience and Learning Curve
A positive developer experience is crucial for project success. Runtimes with intuitive APIs, comprehensive documentation, and helpful tooling can significantly improve developer productivity and satisfaction. A steep learning curve can hinder development speed and introduce unnecessary complexity. Assessing the learning curve and developer experience is important when evaluating the suitability of a runtime for a specific team or project.
Advanced Topics and Use Cases
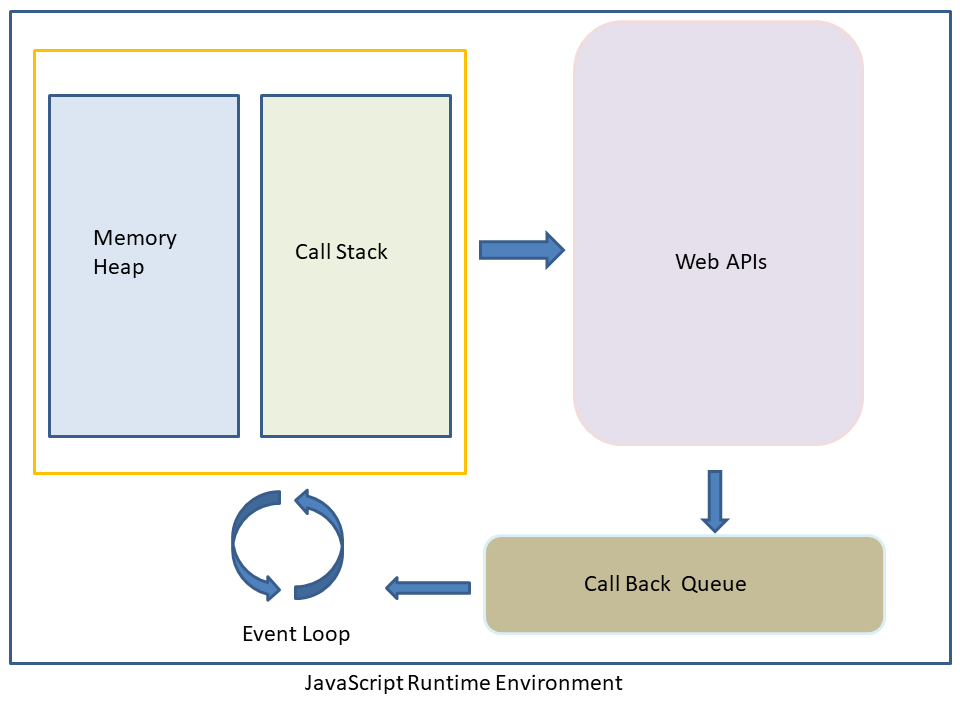
Diving deeper into JavaScript runtime environments reveals a fascinating landscape of applications beyond the browser. Understanding server-side execution, asynchronous handling, and scaling considerations is crucial for developers working on large-scale projects. This section explores these nuances, highlighting the diverse roles these environments play in modern web development.
Server-Side JavaScript
Server-side JavaScript, powered by Node.js and other runtimes, allows JavaScript to execute outside the browser. This is a game-changer for web applications. By processing requests on the server, JavaScript can handle tasks like database interactions, complex calculations, and API calls before sending data back to the client. This significantly enhances responsiveness and allows for more dynamic and feature-rich web experiences.
JavaScript Runtime Environments in Web Development
JavaScript runtime environments are fundamental to web development. They provide the necessary infrastructure for executing JavaScript code, whether in a browser or on a server. They handle everything from parsing the code to managing the execution environment, enabling developers to focus on building applications rather than the underlying complexities. The environment influences how JavaScript interacts with the operating system, other applications, and the broader ecosystem.
Asynchronous Operations
Handling asynchronous operations is a critical aspect of any robust JavaScript application. Different runtime environments offer varying approaches. For example, Node.js utilizes event loops to manage concurrent tasks, making it efficient for handling numerous requests. Other environments may employ different mechanisms, like threads or message queues, to achieve similar results. Understanding how a specific runtime handles asynchronous operations is essential for designing applications that can handle user requests effectively and maintain responsiveness.
Large-Scale Application Implications
Choosing a JavaScript runtime environment for a large-scale application involves careful consideration. Factors such as performance, scalability, and maintainability play a significant role. A well-suited runtime can handle increased traffic and data volumes, allowing the application to grow without performance bottlenecks. Consideration must also be given to the specific needs of the application. If a project involves real-time data streaming, a runtime optimized for this use case would be more appropriate than one primarily designed for simple web page rendering.
Supported Application Architectures
Runtime Environment | Single-Page Applications (SPAs) | Server-Rendered Applications (SRAs) | Microservices | Real-Time Applications |
---|---|---|---|---|
Node.js | Excellent | Excellent | Excellent, often preferred for backend | Good, but other options might be more tailored for specific real-time needs |
Deno | Excellent | Excellent | Good | Good, but other options might be more tailored for specific real-time needs |
V8 (in Browsers) | Excellent, but primarily client-side | Limited, typically client-side rendering | Not ideal | Good for front-end real-time interactions |
This table provides a high-level overview of the suitability of various runtimes for different application architectures. The “Excellent” rating suggests strong support and well-established use cases, while “Good” indicates reasonable support, but potential limitations or specialized considerations.
Security Best Practices in Runtime Environments
JavaScript runtime environments, while powerful, introduce potential security vulnerabilities if not handled meticulously. Understanding these vulnerabilities and implementing robust security measures is crucial for building secure applications. A comprehensive approach involves examining common pitfalls, mitigating them effectively, and employing secure coding practices tailored to the specific runtime environment.Careful consideration of security implications is paramount. A breach in a runtime environment can expose sensitive data, compromise user accounts, or even allow attackers to execute malicious code.
This underscores the importance of proactive security measures throughout the application lifecycle.
Common Security Vulnerabilities
JavaScript runtime environments are susceptible to various security threats. These vulnerabilities often stem from insecure coding practices or limitations within the environment itself. Improper input validation, insufficient access control, and reliance on outdated libraries are common examples. Cross-site scripting (XSS) attacks, injection flaws, and insecure direct object references (IDOR) are all possible outcomes. Careful attention to these areas is essential for creating a secure application.
Mitigation Strategies
Implementing robust security measures is vital for mitigating vulnerabilities. A crucial strategy is thorough input validation. Input should be sanitized and validated to prevent malicious code injection. Proper access control mechanisms are essential to limit access to sensitive data and functionalities. Regular updates to libraries and frameworks are necessary to address known security flaws.
Digging into the best JavaScript runtime environments is fascinating, but lately, I’ve been thinking about the recent news surrounding the end of Pleasanton horse racing. The end of Pleasanton horse racing highlights the unpredictable nature of things, just like how different runtimes can affect your JavaScript code. Ultimately, understanding the strengths and weaknesses of each runtime environment is key to crafting efficient and reliable JavaScript applications.
Secure Coding Practices
Secure coding practices are paramount for developing secure JavaScript applications within various runtime environments. Employing defensive programming techniques is key to reducing vulnerabilities. Using parameterized queries and prepared statements can prevent SQL injection attacks. Input validation, output encoding, and least privilege access control are crucial components of secure coding practices. Secure handling of sensitive data is essential.
Always store passwords using strong hashing algorithms and never store sensitive data in plain text.
Security Implications of Different Runtime Environments
The security implications of different runtime environments vary depending on factors such as the environment’s architecture, features, and the application’s specific requirements. For instance, environments that rely heavily on user-provided data may have higher security concerns. Security best practices must be tailored to the specific environment. Node.js, for example, often requires meticulous handling of asynchronous operations and potentially vulnerable modules.
Choosing the best JavaScript runtime environment can be tricky, with Node.js and Deno often topping the lists. But, the recent devastating Palisades fire, which unfortunately damages or destroys thousands of buildings here , highlights the importance of robust infrastructure, whether for homes or for the efficient running of JavaScript code. Ultimately, selecting the right runtime depends on the specific project needs, but these considerations can help you choose the best environment for your next JavaScript project.
WebAssembly, on the other hand, presents a different set of security challenges related to its interaction with the host environment. Understanding the unique characteristics of each environment is vital for developing secure applications.
Table of Common Security Risks and Mitigation Strategies
Security Risk | Mitigation Strategy |
---|---|
Cross-Site Scripting (XSS) | Input validation, output encoding, Content Security Policy (CSP) |
SQL Injection | Parameterized queries, prepared statements, input validation |
Cross-Site Request Forgery (CSRF) | CSRF tokens, secure headers |
Denial-of-Service (DoS) | Rate limiting, input validation, robust error handling |
Insecure Direct Object References (IDOR) | Proper access control, input validation, secure data handling |
Future Trends and Evolution
JavaScript runtime environments are constantly evolving, driven by the ever-changing needs of web applications and the emergence of new technologies. This dynamic landscape presents both opportunities and challenges for developers, requiring a keen understanding of future trends to effectively leverage the power of these environments. Predicting the precise trajectory of development is inherently difficult, but several factors suggest key directions.Modern runtime environments are evolving beyond simple execution engines.
They are increasingly incorporating features that enhance performance, security, and developer productivity. The rise of serverless computing and the need for more sophisticated tools for managing complex applications are driving this trend.
Emerging Technologies and Their Impact
The integration of WebAssembly is significantly impacting runtime environments. WebAssembly allows developers to write code in languages like C++ and C, which can then be executed within the browser. This dramatically expands the range of possibilities for web applications, enabling the creation of highly performant and feature-rich solutions. Furthermore, the growing use of AI and machine learning in web applications necessitates runtime environments that can handle the computational demands of these algorithms.
This is leading to optimizations in areas like memory management and parallelization.
Performance Enhancements
Runtime environments are continuously striving for greater performance. Optimizations in areas like garbage collection and just-in-time compilation are critical for maintaining smooth user experiences. For example, the use of advanced caching mechanisms is reducing latency and improving responsiveness in applications with high data volume. These performance gains are crucial for handling complex interactions in modern web applications.
Furthermore, the use of parallel processing and multi-threading capabilities is essential to meet the demands of increasingly sophisticated user interfaces and complex algorithms.
Security Considerations
Security is paramount in runtime environments. The need for enhanced protection against vulnerabilities is driving the development of new security features. Runtime environments will likely incorporate more robust sandboxing mechanisms to prevent malicious code from compromising the system. This includes enhanced input validation and code analysis techniques to mitigate vulnerabilities. Moreover, the growing adoption of secure coding practices and static analysis tools is vital in maintaining a secure and trusted runtime environment.
Projected Developments (Next 5 Years)
Year | Area of Development | Description | Example |
---|---|---|---|
2024 | Enhanced WebAssembly Support | Improved performance and integration of WebAssembly modules into runtime environments. | Faster rendering of 3D graphics or scientific simulations within web browsers. |
2025 | AI-Powered Optimization | Runtime environments will utilize AI to dynamically optimize performance based on application behavior. | Automated adjustment of memory allocation based on real-time usage patterns. |
2026 | Advanced Security Features | Inclusion of more sophisticated security features like advanced code analysis and runtime threat detection. | Early detection of malicious code injection attempts before execution. |
2027 | Serverless Integration | Improved support for serverless functions and microservices within runtime environments. | Seamless integration of backend logic into the front-end development process. |
2028 | Quantum Computing Integration (Potential) | Exploration of possibilities for integrating quantum computing capabilities into runtime environments. | (Theoretical) Running complex simulations or data analysis tasks at significantly faster speeds. |
Example Code Snippets (Node.js)
Node.js, built on Chrome’s V8 JavaScript engine, offers a powerful platform for server-side development. Its asynchronous, event-driven architecture enables efficient handling of numerous concurrent requests. This section provides practical examples showcasing fundamental Node.js functionalities.
Basic Node.js Application Structure
A simple Node.js application typically involves creating a JavaScript file (e.g., `app.js`) containing the application logic. The entry point for the application is the `main` function, or often, a specific function or module that executes when the script starts.“`javascript// app.jsconst http = require(‘http’);const hostname = ‘127.0.0.1’;const port = 3000;const server = http.createServer((req, res) => res.statusCode = 200; res.setHeader(‘Content-Type’, ‘text/plain’); res.end(‘Hello World\n’););server.listen(port, hostname, () => console.log(`Server running at http://$hostname:$port/`););“`This example creates an HTTP server.
The `http` module is imported, and the server listens on a specified port and hostname. When a request arrives, it responds with “Hello World.”
File System Operations, Best javascript runtime environments
Node.js offers the `fs` module for interacting with the file system. This module provides functions for reading, writing, and manipulating files.“`javascriptconst fs = require(‘fs’);fs.readFile(‘myFile.txt’, ‘utf-8’, (err, data) => if (err) console.error(“Error reading file:”, err); return; console.log(“File content:”, data););fs.writeFile(‘output.txt’, ‘This is the new content.’, (err) => if (err) throw err; console.log(‘File written successfully’););“`This code snippet demonstrates reading a file (`myFile.txt`) and writing to another file (`output.txt`).
Error handling is included, crucial for robust applications.
Handling Network Requests
The `http` module is essential for handling HTTP requests and responses. It enables creating server-side applications that interact with clients over the network.“`javascriptconst https = require(‘https’);const options = hostname: ‘www.example.com’, port: 443, path: ‘/’, method: ‘GET’;const req = https.request(options, (res) => console.log(‘statusCode:’, res.statusCode); res.on(‘data’, (d) => process.stdout.write(d); ););req.on(‘error’, (e) => console.error(e););req.end();“`This example utilizes the `https` module to make a GET request to `www.example.com`.
The response is streamed, allowing handling of large responses efficiently.
Choosing the best JavaScript runtime environment really depends on the project, but Node.js is often a great choice for server-side applications. While the NFL’s recent drama, like the 49ers’ response to the latest controversy , is definitely captivating, understanding the strengths of different runtimes is crucial for developers. Ultimately, the right runtime streamlines the development process and helps produce high-quality results.
Asynchronous Nature
Node.js’s asynchronous nature is vital for performance. It allows handling multiple requests concurrently without blocking the main thread.“`javascriptconst readFile, writeFile = require(‘fs/promises’);async function processFiles() try const data1 = await readFile(‘file1.txt’, ‘utf-8’); const data2 = await readFile(‘file2.txt’, ‘utf-8’); const combinedData = data1 + data2; await writeFile(‘combined.txt’, combinedData); console.log(‘Files processed successfully’); catch (error) console.error(‘Error:’, error); processFiles();“`This code uses `async/await` for cleaner asynchronous code.
The `processFiles` function reads two files, combines their content, and writes the result to a new file, demonstrating asynchronous operations in Node.js.
Creating a Simple Node.js Application
Step | Description |
---|---|
1. | Install Node.js and npm (Node Package Manager). |
2. | Create a new JavaScript file (e.g., `app.js`). |
3. | Import necessary modules (e.g., `http`, `fs`). |
4. | Implement the core logic of your application (e.g., handling requests, file operations). |
5. | Use the `http` module or other appropriate methods to start the server. |
6. | Run the application using `node app.js`. |
These steps Artikel the essential steps for creating a Node.js application.
Example Code Snippets (Deno): Best Javascript Runtime Environments
Deno, a modern JavaScript runtime, offers a compelling alternative to Node.js, particularly for its focus on security and modularity. This section delves into practical Deno examples, demonstrating its key features and comparing its coding style with Node.js. Deno’s built-in tools and approach to file handling and modules are key differentiators.
Using Deno’s `-l` Flag (Line Interface)
Deno’s `-l` flag allows running code directly from the command line. This is a powerful feature for quick prototyping and testing.“`bashdeno run -l –allow-read=./input.txt my_script.ts“`This command runs `my_script.ts`, enabling read access to the `input.txt` file. The `–allow-read` flag is crucial for file system interaction, granting specific permissions. This example highlights Deno’s principle of explicit permissions, enhancing security by restricting access.
File System Interaction in Deno
Deno’s file system interaction is straightforward and safe. It uses the `Deno.readTextFile` function, which takes a file path as an argument.“`typescriptimport readTextFile from “https://deno.land/[email protected]/fs/mod.ts”;async function main() const filePath = “./my_file.txt”; const fileContent = await readTextFile(filePath); console.log(fileContent);main();“`This example reads the contents of `my_file.txt` and prints them to the console. The `async` and `await` s are standard for handling asynchronous operations.
Importantly, the example utilizes a module from the Deno standard library (`https://deno.land/[email protected]/fs/mod.ts`), which is a crucial part of Deno’s modular approach.
Using Deno’s Built-in Modules
Deno’s standard library provides a rich set of modules for common tasks. This example uses the `colors` module to add color to console output.“`typescriptimport red from “https://deno.land/[email protected]/fmt/colors.ts”;console.log(red(“This text is red!”));“`This snippet demonstrates how to import and use the `red` function from the `colors` module to display colored output in the console. This showcases the ease of using pre-built, well-documented modules.
Comparison of Node.js and Deno Code Structures
This table compares the code structures for similar functionalities in Node.js and Deno, highlighting differences in file handling and module import syntax.
Feature | Node.js | Deno |
---|---|---|
File System Reading | `fs.readFileSync` (synchronous) or `fs.readFile` (asynchronous) | `Deno.readTextFile` (asynchronous) |
Module Import | `require(‘module-name’)` | `import moduleName from ‘module-path’` |
Error Handling | Error handling often embedded within callbacks or promises | `try…catch` blocks and asynchronous handling |
This comparison underscores Deno’s clear separation of synchronous and asynchronous operations and its preference for asynchronous programming models, which is consistent with its modern approach.
Conclusive Thoughts
In conclusion, the landscape of JavaScript runtime environments is rich and dynamic. From the seasoned veteran Node.js to the promising newcomer Deno, developers have a plethora of options for executing JavaScript code effectively. Careful consideration of performance, security, and developer experience is crucial when selecting the right environment for your project. This comprehensive guide provides a foundation for making informed decisions, empowering developers to choose the optimal solution for their specific needs.
The future looks bright, with continued innovation in this field.