Resolve Cannot Find Symbol Error Java A Comprehensive Guide
Resolve cannot find symbol error java plagues Java developers, often leading to frustrating debugging sessions. This comprehensive guide dives deep into understanding this common error, dissecting its causes, and providing effective troubleshooting techniques. We’ll explore different scenarios where this error arises, offering clear explanations and practical examples to help you resolve these issues swiftly and efficiently.
From identifying missing symbols to correcting the error, this guide provides a structured approach to tackling this prevalent Java problem. We’ll cover crucial aspects like proper variable naming, package structures, and import statements, ensuring a solid foundation in preventing similar errors in the future.
Understanding the Error
The “resolve cannot find symbol error” in Java is a common compiler error that arises when the Java compiler encounters a reference to a variable, method, class, or interface that it cannot locate within the current scope or any imported packages. This often indicates a typo, a missing import statement, or a problem with the project structure. Accurately diagnosing this error is crucial for efficient debugging and swift resolution.The error stems from the compiler’s inability to link the requested identifier to its corresponding declaration.
This is typically caused by misspellings, incorrect capitalization, or issues with the Java file structure. Furthermore, missing or incorrect import statements, which prevent the compiler from accessing necessary classes or packages, can also lead to this error.
Common Causes
The “resolve cannot find symbol” error frequently results from a combination of factors, including:
- Incorrect Identifiers: Typos or incorrect capitalization in variable, method, or class names. For example, referencing “myVarriable” when the correct name is “myVariable”.
- Missing Import Statements: The compiler needs to know where to find classes from external libraries or packages. If an import statement is missing, the compiler will not be able to locate the required class.
- Incorrect Package Structure: Problems with the project structure, including incorrect file paths or package declarations that do not match the actual file location.
- Incorrect Class or Method Usage: A method call that doesn’t exist within the class, or a variable access that isn’t declared.
- Cyclic Dependencies: In some cases, an error can occur due to dependencies that form a loop. This can lead to circular references, which the compiler cannot resolve.
Reproducing the Error
Here are examples demonstrating how to reproduce the “resolve cannot find symbol” error in various situations:
- Typo in Variable Name:
“`java
public class Example
public static void main(String[] args)
int myVarriable = 10; //Typo
System.out.println(myVarriable);That pesky “resolve cannot find symbol” error in Java can be a real headache. Sometimes, it’s a simple typo, but other times, it’s a deeper issue. Switching to a more robust hosting environment can actually indirectly help. For instance, if you’re looking for the best nodejs hosting platforms, best nodejs hosting platforms often offer more predictable environments, making debugging less frustrating.
Ultimately, thorough code reviews and meticulous attention to detail are key to resolving the “resolve cannot find symbol” error.
“`
This code will produce the error because the compiler cannot find a variable named “myVarriable”. - Missing Import Statement:
“`java
public class Example
public static void main(String[] args)
java.util.Date date = new java.util.Date(); //missing import
System.out.println(date);“`
The compiler cannot find the `Date` class without the proper import statement. - Incorrect Class Usage:
“`java
public class Example
public static void main(String[] args)
String str = “Hello”;
str.print(); // print() is not a method of String“`
This example will result in an error as the String class doesn’t have a `print` method.
Importance of Error Messages
Understanding the error message is vital for efficient debugging. The error message typically points to the line of code causing the problem, often including the specific identifier that cannot be resolved. Careful examination of the context surrounding the error message can help to quickly isolate the source of the issue.
Troubleshooting Table
Error Message | Possible Cause | Solution | Example |
---|---|---|---|
cannot find symbol – variable x | Typo in variable name, missing declaration | Check the spelling of ‘x’, ensure it’s declared | “`javaint x = 10; //missing declarationSystem.out.println(x);“` |
cannot find symbol – method print | Missing import statement or incorrect method name | Add import statement or correct method name | “`javaimport java.io.*;// …FileWriter writer = new FileWriter(“file.txt”);writer.print(“hello”); //correct usage“` |
cannot find symbol – class MyClass | Missing import statement, incorrect package name | Add import statement or fix package name | “`javaimport mypackage.MyClass; //missing import// …MyClass obj = new MyClass();“` |
Identifying the Missing Symbol: Resolve Cannot Find Symbol Error Java
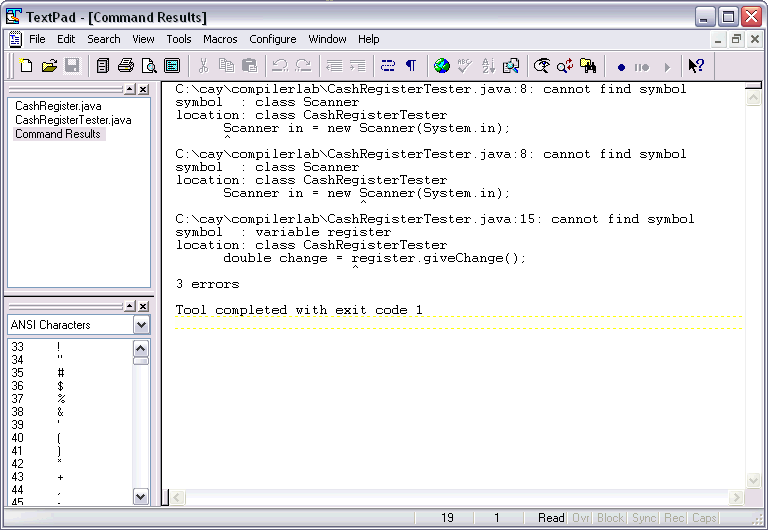
The “cannot find symbol” error in Java is a common headache for developers. It arises when the compiler encounters a reference to a variable, method, or class that doesn’t exist in the current scope. This error message, while seemingly straightforward, can be tricky to diagnose, particularly when dealing with complex codebases. Pinpointing the exact source of the problem requires a methodical approach to understanding the code’s structure and the compiler’s perspective.Understanding the context of the error is crucial.
The error is not always a simple oversight; it can indicate deeper issues in your program’s logic or structure. Knowing where the error occurs, and what code element is missing, is the key to fixing it. This guide will delve into the common types of missing symbols, providing practical examples and highlighting the importance of proper naming conventions to help you effectively identify and resolve these errors.
Common Types of Missing Symbols
Java relies on a structured hierarchy of elements. Variables, methods, and classes are all part of this structure, and they must be declared and defined correctly for the compiler to understand them.
- Missing Variables: Variables hold data values. If you try to use a variable that hasn’t been declared, the compiler will flag it as a missing symbol. This is often caused by typos in the variable name or forgetting to declare the variable before using it.
- Missing Methods: Methods define actions or tasks. If a method is referenced but hasn’t been defined, the compiler will report the “cannot find symbol” error. This could stem from incorrect method names or mismatched parameter lists in the method call. Example: Attempting to invoke a method with an incorrect number or type of arguments.
- Missing Classes: Classes are blueprints for creating objects. A reference to a class that doesn’t exist in the project or is not imported correctly results in this error. For instance, trying to use a class from a library without including the necessary import statement.
Identifying Missing Variables
Variables are essential building blocks in Java programs. Their names must adhere to specific conventions for clarity and maintainability.
- Typographical Errors: A simple typo in a variable name can lead to the compiler failing to recognize it. Always double-check the spelling when referencing variables.
- Scope Issues: Variables have scopes, meaning they are only accessible within a specific block of code. If you try to access a variable outside its scope, you’ll get a “cannot find symbol” error. Consider whether the variable is declared in a parent scope, a nested scope, or not at all.
- Incorrect Naming Conventions: While Java allows flexibility, adhering to naming conventions helps prevent errors. Variable names should start with a lowercase letter and use camel case for multi-word names (e.g., `userName`, `customerAddress`).
Identifying Missing Methods and Classes
Methods and classes are critical for structuring and organizing Java programs.
- Incorrect Method Names: Carefully review the method signature (name and parameters) to ensure it matches the definition in the code.
- Missing Import Statements: If you are using a class from a different package, you need an import statement. Without it, the compiler cannot locate the class. Using the `import` is crucial to bridge the gap between the compiler and the referenced class.
- Incorrect Class Names: Verify the class name you’re referencing matches the class’s actual name in the project or the library you’re using. Be meticulous when comparing names.
Importance of Variable Naming Conventions
Consistent and descriptive variable names are essential for readability and maintainability.
Inconsistencies in variable names can contribute to errors. Adhering to naming conventions helps prevent errors by making the code more self-documenting and easier to understand. The compiler doesn’t enforce these conventions, but following them reduces the likelihood of errors and improves code quality.
Comparison of Symbol Types
Symbol Type | Example | Error Description | Solution |
---|---|---|---|
Variable | int age; |
The variable ‘ages’ is not declared in this scope. | Correct the typo: int age; |
Method | public void displayMessage() |
Cannot find symbol – method displayMessage2() | Ensure the method name is correct: public void displayMessage() |
Class | import java.util.Scanner; |
Cannot find symbol – class Scanner | Ensure the import statement is correct and the class exists: Add the import statement if missing. Verify the class name. |
Troubleshooting Techniques
The “cannot find symbol” error in Java is a common frustration, often stemming from seemingly innocuous typos or overlooked details. This section provides practical strategies to pinpoint and resolve these errors, empowering you to efficiently debug your Java code.Effective debugging relies on methodical approaches.
By systematically examining code segments and utilizing available tools, you can isolate the root cause of the “cannot find symbol” error and implement a solution swiftly.
Locating Missing Symbols in Code
Understanding the context of the error message is crucial. The error message often points to the specific line of code where the symbol is missing. This provides a starting point for your investigation. Carefully review the code surrounding the error location, paying attention to variable declarations, method calls, and import statements.
Using Debugging Tools
Integrated Development Environments (IDEs) offer powerful debugging tools that help trace the flow of execution within your code. These tools allow you to step through code line by line, inspect variables, and understand how your program interacts with the missing symbol. Utilizing breakpoints, watch windows, and the ability to examine the program’s state are essential for effective debugging.
Analyzing Code Segments
A systematic approach to analyzing code segments is essential. Start by identifying the specific symbol that is missing. Examine the surrounding code to determine if the symbol is declared or defined in the current scope. Check if the symbol’s name is correctly spelled and capitalized, ensuring it matches the declaration. Verify the appropriate import statements are present if the symbol belongs to a different class.
Look for any potential syntax errors that might be hindering the compiler from recognizing the symbol.
So, you’re wrestling with a “cannot find symbol” error in your Java code? It’s a common frustration, but often the solution is surprisingly straightforward. Sometimes, it’s a simple typo, or a missing import. To distract from your coding woes, you might want to check out some of the incredible birdwatching opportunities in California’s Central Valley, like the vast grasslands and riparian areas perfect for spotting a diverse array of species.
great places to see birds in californias central valley. Then, return to your code and double-check your class names and method signatures. With a bit of attention to detail, you’ll have that error resolved in no time.
Verifying Spelling and Capitalization
Errors in spelling or capitalization are frequent sources of the “cannot find symbol” error. Manually verify that the symbol’s name, as it appears in your code, perfectly matches the name of the declared variable, method, or class. Case sensitivity is crucial in Java; a single incorrect case can lead to the error. Use the IDE’s auto-completion features to assist in checking the correct spelling and capitalization.
Utilizing Code Editor/IDE Features
Modern IDEs provide helpful features for locating missing symbols. The IDE’s code completion feature can often identify the missing symbol, if it exists. Furthermore, the IDE’s search function can help locate the declaration of the missing symbol within the project. Use these tools effectively to enhance your debugging process. Look for error highlighting and suggestions that the IDE might offer.
These visual cues can often pinpoint the issue.
Correcting the Error
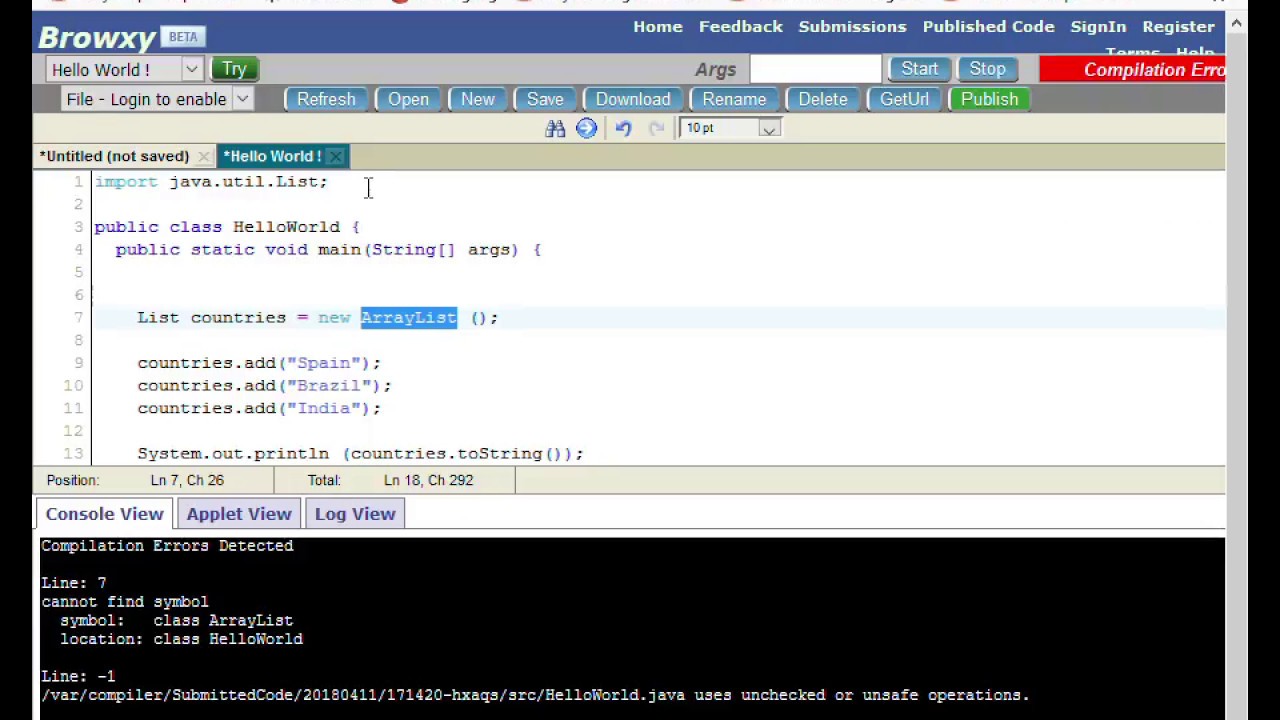
The “cannot find symbol” error in Java often stems from a missing or incorrectly used identifier. This identifier could be a variable, method, class, or even an import statement. Understanding the cause of the error is crucial; now we’ll focus on how to effectively resolve it by addressing the missing symbol directly within the code.Correcting this error involves a multifaceted approach.
It’s not just about adding the symbol; it’s about ensuring its proper integration into the existing code structure, including package management and import statements. Understanding the proper syntax and scope is essential to avoid future issues.
Trying to figure out a “resolve cannot find symbol error” in Java can be frustrating. It’s often a simple typo, but sometimes you need a fresh perspective. For instance, have you considered viewing the serene beauty of Japan? A captivating photo exhibit at Hakone, photo exhibit at Hakone shows Japan through a contemplative lens , might offer just that.
Maybe stepping back and appreciating the details, like the precision in Japanese artistry, can help you spot the missing piece in your Java code. Ultimately, sometimes a change of scenery can help resolve those coding conundrums.
Adding the Missing Symbol
To correct the “cannot find symbol” error, you need to identify the missing identifier (e.g., a class, method, or variable) and add it to the code. This often involves declaring the missing element.
- Example 1: Missing Class
If you’re using a class that hasn’t been imported, you must import it first. For instance, if you want to use the
java.util.ArrayList
class, you need the import statement.import java.util.ArrayList; public class MyClass public static void main(String[] args) ArrayList
myList = new ArrayList<>(); // Now compiles without errors myList.add("Hello"); - Example 2: Missing Method
If a method isn’t defined within the current class or in a correctly imported class, you’ll get the error. Consider this scenario:
public class MyClass public static void main(String[] args) String message = "Hello"; System.out.println(capitalize(message)); // Error: cannot find symbol // Correct implementation: public static String capitalize(String str) return str.toUpperCase();
The
capitalize
method needs to be defined within the class for the code to compile correctly.
Proper Syntax
Correct syntax is paramount for avoiding the “cannot find symbol” error. The syntax of the missing symbol must match the expected format in the Java language.
- Import Statements: Ensure the import statements are accurate. For example, if you’re using a class from a package, use the fully qualified package name in the import statement.
- Method Calls: Verify the correct number and types of arguments for a method call. Incorrect argument types or missing arguments can trigger the error.
- Variable Usage: Ensure that variables are declared before they are used, and that their types are consistent with their intended usage.
Package Structure and Imports
The structure of your project’s packages directly impacts whether the compiler can find the required classes.
- Clear Structure: Maintain a well-organized package structure. This makes it easier to locate classes and ensures correct import statements.
- Correct Imports: Ensure that the correct import statements are used to access classes from other packages. Use fully qualified names where needed. A well-defined import is vital.
Scope
Understanding the scope of a symbol (class, method, or variable) is crucial.
- Visibility: Ensure the symbol is visible within the part of the code that uses it. If a method or variable is private, you won’t be able to access it from outside the class where it is declared.
- Block Scope: Be mindful of block scope. Variables declared within a block (e.g., inside an
if
statement) are only accessible within that block.
Best Practices for Preventing Future Errors
- Thorough Code Reviews: Have someone else review your code to catch potential errors like missing imports or incorrect syntax.
- Comprehensive Testing: Thorough testing, including unit tests, helps to uncover errors early on.
- Consistent Coding Style: Adhering to a consistent coding style helps maintain readability and reduces errors.
Illustrative Examples
The “cannot find symbol” error in Java often arises from subtle mistakes in your code. Understanding how these errors manifest in various scenarios is crucial for effective debugging. These examples will demonstrate how different types of missing symbols lead to this error and illustrate the solutions.
Missing Method
The “cannot find symbol” error can occur when a method you’re trying to call doesn’t exist in the class you’re working with. This often stems from typos, incorrect capitalization, or simply forgetting to define the method.
public class MyClass
public void myMethod()
System.out.println("Hello from myMethod!");
public static void main(String[] args)
MyClass obj = new MyClass();
obj.myMetod(); // Incorrect method name
In this example, the `main` method attempts to call the `myMetod` method, which doesn’t exist in the `MyClass` class. This results in a “cannot find symbol” error on the `obj.myMetod();` line.
// Corrected code
public class MyClass
public void myMethod()
System.out.println("Hello from myMethod!");
public static void main(String[] args)
MyClass obj = new MyClass();
obj.myMethod(); // Correct method name
The corrected code uses the correct method name, `myMethod()`, eliminating the error.
Undeclared Variable
A “cannot find symbol” error can also occur when a variable is used in a program but hasn’t been declared within the current scope.
public class VariableExample
public static void main(String[] args)
int x = 10;
System.out.println(y); // y is not declared
Here, the program attempts to print the value of `y` without declaring it. This results in a compilation error because `y` is undefined within the `main` method’s scope.
// Corrected code
public class VariableExample
public static void main(String[] args)
int x = 10;
int y = 20; // Declare y
System.out.println(y);
The corrected code declares the `y` variable before using it, resolving the error.
Missing Import Statement
The compiler might not be able to find a class if the necessary import statement isn’t included.
public class ImportExample
public static void main(String[] args)
java.util.Date date = new java.util.Date(); // Missing import
System.out.println(date);
This example tries to use the `Date` class from the `java.util` package without importing it.
// Corrected code
import java.util.Date; // Import the Date class
public class ImportExample
public static void main(String[] args)
Date date = new Date();
System.out.println(date);
The corrected code includes the `import java.util.Date;` statement, allowing the compiler to locate the `Date` class.
Method Not Declared in Class
Sometimes, the “cannot find symbol” error points to a method that is not defined within the class where it’s called.
public class MethodExample
public static void main(String[] args)
AnotherClass another = new AnotherClass();
another.anotherMethod(); // Method not declared in MethodExample
This example calls `anotherMethod()` from `AnotherClass`, but the method is not present in `MethodExample`.
// Correct Class
public class AnotherClass
public void anotherMethod()
System.out.println("Hello from anotherMethod!");
// Correct Usage
public class MethodExample
public static void main(String[] args)
AnotherClass another = new AnotherClass();
another.anotherMethod();
The correction involves defining `anotherMethod()` in `AnotherClass` and ensuring the class is used correctly in `MethodExample`.
Error Prevention
The “resolve cannot find symbol” error in Java often stems from subtle coding mistakes, making prevention a crucial aspect of robust development. Proactive measures can significantly reduce debugging time and improve code quality. Careful attention to detail and consistent coding practices are essential to avoid this common error.
Careful Coding Practices
Implementing consistent and well-defined coding standards minimizes the risk of introducing errors. This includes adhering to naming conventions, using meaningful variable names, and writing clear and concise code. Employing these practices fosters maintainability and readability, both of which are key to avoiding the “cannot find symbol” error.
Verifying Code Structure and Syntax
Thorough code verification is paramount in preventing errors. Employing tools such as linters and static analysis tools helps detect potential syntax issues and structural problems before compilation. These tools identify discrepancies, including missing semicolons, incorrect variable declarations, and inappropriate data types, which can lead to the “resolve cannot find symbol” error.
Managing Project Dependencies and Libraries
Proper management of project dependencies is vital. Incorrect or outdated libraries can introduce conflicts that result in the “resolve cannot find symbol” error. Using dependency management tools like Maven or Gradle ensures that all dependencies are correctly specified and resolved. These tools also help prevent conflicts among libraries and their versions.
Using Code Review to Prevent Errors, Resolve cannot find symbol error java
Code reviews offer a valuable opportunity for identifying potential errors early in the development process. By having peers examine code, developers can catch issues before they impact the overall project. A structured code review process, with specific steps and guidelines, can significantly reduce errors.
Detailed Code Review Process
A structured code review process can proactively prevent the “resolve cannot find symbol” error. This process ensures that the code is reviewed comprehensively, identifying potential errors before they impact the project.
- Review Objective: The primary objective is to identify and address potential issues that might lead to the “resolve cannot find symbol” error, such as incorrect variable declarations, missing imports, or incompatible types.
- Code Understanding: The reviewer should thoroughly understand the code’s purpose and logic. They should understand how the code interacts with other parts of the application.
- Syntax and Structure Check: Pay close attention to the code’s syntax and structure. Look for missing semicolons, incorrect variable declarations, or inappropriate use of data types. This helps identify potential “resolve cannot find symbol” errors.
- Dependency and Library Verification: Verify that all necessary libraries and dependencies are correctly included and properly integrated into the project. Ensure that there are no conflicts between library versions.
- Naming Convention Review: Verify adherence to naming conventions. Ensure that variable and method names are clear, concise, and follow established conventions. Inconsistent naming conventions can lead to misinterpretations.
- Potential Error Cases: Discuss potential error scenarios and how the code handles them. This review helps identify situations where the “resolve cannot find symbol” error might arise.
- Feedback and Discussion: Provide constructive feedback to the author. Discuss any identified issues, propose solutions, and collaborate to address them effectively.
Last Point
In conclusion, resolving the “cannot find symbol” error in Java is achievable with a methodical approach. By understanding the error’s origins, identifying the missing symbol, and employing effective troubleshooting techniques, you can efficiently fix these issues and enhance your Java programming skills. The examples and detailed explanations in this guide will equip you with the knowledge to prevent these errors in future projects and ensure smooth Java development.