Calculate Time Difference in Python A Deep Dive
Calculate time difference in Python is a crucial skill for any programmer. From tracking script execution times to analyzing user behavior, understanding time differences is fundamental. This comprehensive guide delves into the intricacies of calculating time differences in Python, exploring various methods and considerations.
We’ll cover fundamental Python libraries like `datetime` and `time`, demonstrate calculating differences between dates and times, and highlight the importance of time zones. We’ll also examine advanced techniques, error handling, and practical examples, making this guide a practical resource for any Python developer.
Introduction to Time Difference Calculation in Python: Calculate Time Difference In Python
Calculating time differences is a fundamental task in many Python applications, enabling the precise measurement of durations between events. This process is crucial for tasks ranging from simple logging to complex scientific simulations. Understanding how to perform these calculations accurately and efficiently is vital for reliable program execution and meaningful analysis of data.Python provides robust tools for working with dates and times, allowing developers to handle a wide variety of time-related problems.
The `datetime` module, in particular, offers classes for representing dates, times, and time intervals, making time difference calculations straightforward. This article will explore the core concepts, common use cases, and essential data types for effective time difference calculation in Python.
Importance of Time Difference Calculations
Time difference calculations are indispensable in a multitude of applications. From tracking user engagement on websites to analyzing stock market fluctuations, accurately determining time intervals is essential for understanding patterns and trends. In scientific research, precisely measuring time differences is critical for experiments and data analysis.
Common Use Cases
Numerous applications rely on calculating time differences. These include:
- Performance Monitoring: Analyzing the time taken for different operations within a program to identify bottlenecks and optimize code efficiency. For example, measuring the time to execute a database query or process a large dataset.
- Event Logging: Recording the time of occurrence for specific events, such as user logins, errors, or system updates. This allows for identifying trends and diagnosing problems.
- Financial Analysis: Determining the duration of investments, calculating interest rates, or analyzing market fluctuations over time.
- Scientific Simulations: Modeling physical phenomena that involve time-dependent processes. This is particularly important in fields like physics, chemistry, and biology.
Fundamental Concepts and Terminology
The core concept in time difference calculation revolves around representing dates and times in a structured format and then measuring the interval between them. Understanding the following terms is critical:
datetime: A Python object that encapsulates a specific date and time. timedelta: A Python object that represents a duration of time, such as the difference between two datetime objects.
Data Types for Representing Time
Different data types are used to represent time in Python. This table summarizes the common types and their roles:
Data Type | Description |
---|---|
datetime |
Represents a specific point in time, including year, month, day, hour, minute, second, and microsecond. |
timedelta |
Represents a duration of time, such as the difference between two datetime objects. It does not represent a specific point in time. |
Python Libraries for Time Handling
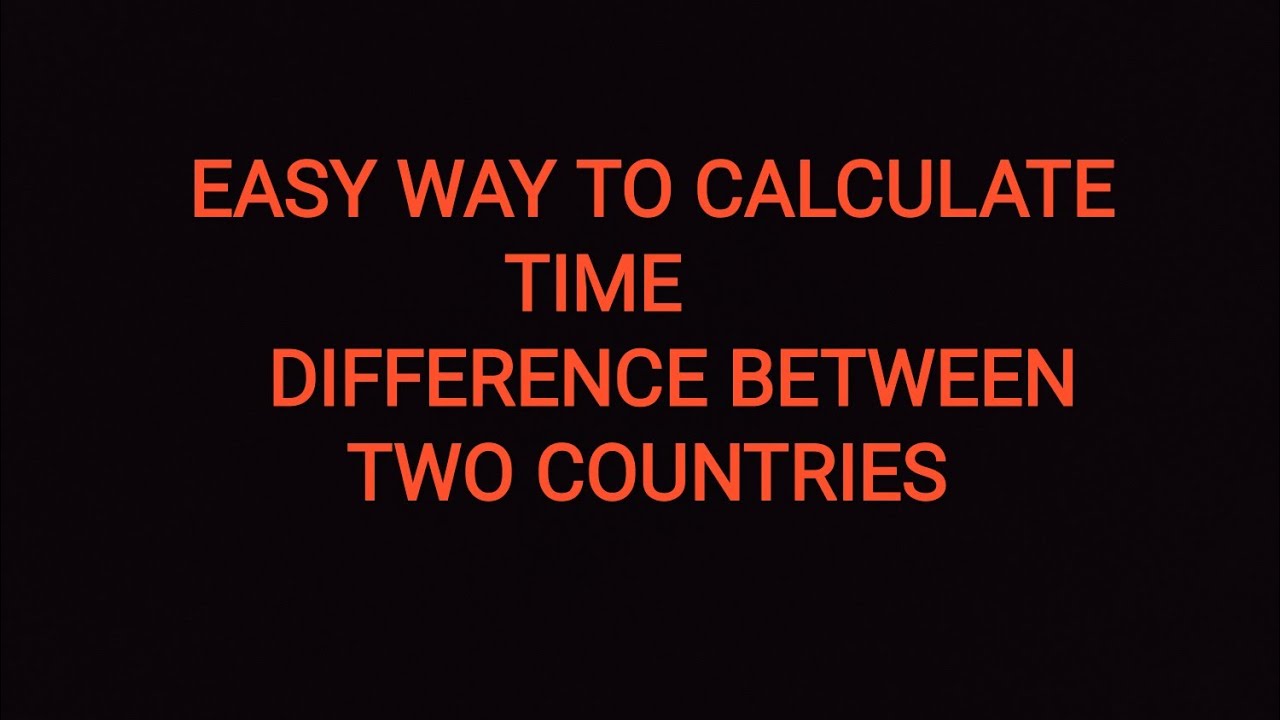
Python offers robust tools for working with dates and times, crucial for tasks involving time difference calculations. These libraries provide a structured and efficient way to represent and manipulate timestamps, facilitating complex operations like calculating time intervals and handling time zones. Understanding these libraries is essential for building applications that require precise time management.The `datetime` and `time` modules are fundamental for time-related tasks in Python.
They provide different levels of functionality, with `datetime` focusing on date and time objects, and `time` concentrating on time-related metrics. Understanding their nuances and how to combine them is key to efficiently handling time differences in various applications.
The `datetime` Module
The `datetime` module is designed for working with dates and times. It’s particularly useful when dealing with calendar-related operations, such as date arithmetic, formatting, and comparisons. The `datetime` object combines both date and time components, offering a comprehensive representation.
The `time` Module
The `time` module provides functions for interacting with the system’s time. It focuses primarily on time-related aspects like obtaining the current time, measuring execution time, and working with time in a more granular format. Unlike `datetime`, it does not incorporate date information.
The `timedelta` Object, Calculate time difference in python
The `timedelta` object, a part of the `datetime` module, is a powerful tool for representing durations or differences between dates and times. It’s essential for calculations involving time intervals, enabling operations like adding or subtracting time from a given date or time.
Usage of the `time` Module
The `time` module allows accessing the current time in various formats. Functions like `time.time()` return the current time as a floating-point number representing seconds since the epoch. `time.localtime()` converts this timestamp into a time tuple representing local time. This is beneficial for applications needing to track execution time or perform operations based on the system’s current time.
Usage of the `datetime` Module
The `datetime` module offers comprehensive date and time manipulation. Creating `datetime` objects involves specifying year, month, day, hour, minute, second, and microsecond. Methods like `datetime.now()` provide the current date and time.
Comparing `datetime` and `time`
The `datetime` module offers a more comprehensive approach to date and time management, including both date and time information. The `time` module focuses on time-related aspects without the date component. For tasks involving both date and time, `datetime` is preferable. If the application primarily needs to track elapsed time, `time` provides a more focused and efficient method.
Working with Time Zones
Python’s `datetime` module supports time zones through the `pytz` library. Using `pytz` allows representing and converting times across different time zones, crucial for applications requiring global time awareness.
Creating `datetime` and `timedelta` Objects
Method | Description | Example |
---|---|---|
`datetime.datetime.now()` | Returns the current date and time. | `datetime.datetime.now()` |
`datetime.datetime(year, month, day, hour, minute, second, microsecond)` | Creates a `datetime` object with specific values. | `datetime.datetime(2024, 10, 27, 10, 30, 0)` |
`datetime.timedelta(days, seconds, microseconds, milliseconds, minutes, hours, weeks)` | Creates a `timedelta` object representing a time difference. | `datetime.timedelta(days=1, hours=2)` |
Calculating Time Differences
Calculating time differences is a fundamental task in many applications, from tracking project durations to analyzing historical data. Python’s `datetime` module provides robust tools for handling dates and times, making it easy to determine the interval between two points in time. This section will delve into the practical aspects of calculating time differences using Python’s `datetime` module and `timedelta` objects, including handling time zones and potential errors.
Calculating Time Differences Between Datetime Objects
To calculate the difference between two `datetime` objects, we utilize the `timedelta` object. This object represents a duration, not a specific point in time. Subtracting one `datetime` object from another yields a `timedelta` object, holding the difference in time.
Example:
import datetime start_time = datetime.datetime(2024, 10, 26, 10, 0, 0) end_time = datetime.datetime(2024, 10, 26, 12, 30, 0) time_difference = end_time - start_time print(time_difference)
This code snippet calculates the time difference between `start_time` and `end_time`. The output will be a `timedelta` object, providing the duration in days, seconds, and other units.
Using Timedelta Objects for Calculations
The `timedelta` object offers methods to extract specific components of the time difference. This allows for precise control over how the difference is presented.
Example:
import datetime time_difference = datetime.timedelta(days=2, hours=3, minutes=30) days = time_difference.days seconds = time_difference.total_seconds() hours = time_difference.seconds // 3600 minutes = (time_difference.seconds % 3600) // 60 print(f"Days: days") print(f"Seconds: seconds") print(f"Hours: hours") print(f"Minutes: minutes")
This example demonstrates extracting days, seconds, hours, and minutes from a pre-defined `timedelta` object.
Time Difference Calculations Table
Component | Calculation | Example |
---|---|---|
Days | `time_difference.days` | If `time_difference` represents 2 days, 3 hours, the result is 2. |
Seconds | `time_difference.total_seconds()` | If `time_difference` represents 2 days, 3 hours, the result is the total time in seconds. |
Hours | `time_difference.seconds // 3600` | The integer division (`//`) extracts the whole number of hours. |
Minutes | `(time_difference.seconds % 3600) // 60` | The modulo operator (`%`) and integer division (`//`) extract the remaining minutes. |
Converting Time Units
Converting between time units (e.g., seconds to minutes) is straightforward using the appropriate mathematical operations. Remember to consider potential fractional components.
Handling Time Zones
When dealing with time zones, ensure that the `datetime` objects are properly aware of the time zone. Use the `pytz` library for accurate time zone handling.
Error Handling
Always validate inputs to prevent unexpected errors. Check for invalid date formats, missing data, or potential `ValueError` exceptions. Robust error handling ensures your calculations are reliable.
Handling Time Zones
Time is a crucial element in any application involving timestamps and time-based computations. However, the way time is perceived and represented varies across the globe due to time zones. Ignoring time zones can lead to significant inaccuracies, particularly when dealing with international operations or data collected from different locations. This section delves into the intricacies of handling time zones in Python for accurate time difference calculations.Time zone awareness is critical for accurate time difference calculations.
Simply treating time as a numerical value without considering the underlying time zone can lead to errors in determining the actual elapsed time between events occurring in different locations.
Understanding Time Zones in Python
Python’s `datetime` module, while powerful, lacks built-in time zone support. The `pytz` library provides a solution to this, enabling the representation and handling of time zones. This library provides comprehensive data for various time zones around the world. Using `pytz`, Python can correctly account for daylight saving time (DST) transitions, which significantly impact time calculations.
Working with pytz
The `pytz` library is essential for handling time zones effectively in Python. It allows you to represent and manipulate time zone information accurately. This involves using `pytz.timezone` to obtain a specific time zone object. It’s crucial to ensure that all timestamps are associated with a specific time zone.
Converting Between Time Zones
Converting between time zones is vital when dealing with data from different locations. Using the `astimezone` method, you can convert a `datetime` object from one time zone to another. This ensures the correct time representation when comparing events across various geographical regions. This is demonstrated in the examples below.
Calculating Time Differences Across Time Zones
To calculate time differences accurately across time zones, ensure all timestamps are aware of their respective time zones. This is best done by using `pytz.timezone` to create time zone objects.“`pythonimport pytzfrom datetime import datetime# Example of calculating time difference across time zoneseastern = pytz.timezone(‘US/Eastern’)pacific = pytz.timezone(‘US/Pacific’)eastern_dt = eastern.localize(datetime(2024, 10, 27, 10, 0, 0))pacific_dt = pacific.localize(datetime(2024, 10, 27, 7, 0, 0))time_diff = eastern_dt – pacific_dtprint(time_diff)“`This example demonstrates how to correctly create `datetime` objects with time zone awareness.
The `time_diff` represents the difference in time between the two events in a specific time zone.
The Role of Time Zone Awareness
Accurate time difference computations rely on proper time zone handling. Ignoring time zones leads to inaccurate results, especially when dealing with international data or operations. Inaccurate time differences can affect scheduling, reporting, and analysis. For instance, a business dealing with customers globally must consider time zone differences to avoid conflicts or misinterpretations.
Comparison of Time Zone Handling Approaches
Approach | Description | Accuracy | Complexity |
---|---|---|---|
Naive Timestamps | Treats timestamps as numerical values without time zone information. | Inaccurate | Simple |
pytz Library | Uses time zone objects to maintain awareness of time zones, crucial for accurate calculations. | Accurate | Moderate |
The table highlights the importance of using `pytz` for accurate time zone handling in Python. Naive approaches can lead to significant errors, while the `pytz` library provides a robust and accurate way to manage time zone data.
Practical Applications and Examples
Time difference calculations are fundamental in various domains, from tracking program execution time to analyzing user behavior. Understanding how to efficiently calculate and interpret these differences unlocks insights into performance, efficiency, and user experience. This section dives into practical applications with detailed examples.Calculating time differences is crucial for performance analysis in software development. Whether tracking the time taken by a script or analyzing delays in a process, accurate timing helps in optimizing performance and identifying bottlenecks.
The examples below demonstrate these applications.
Calculating time differences in Python is a pretty straightforward task, often useful for various applications. For instance, as they take office, new Bay Area congressmembers, as reported in this article as they take office new bay area congressmembers pledge to bring bold solutions and protect their diverse constituencies , are likely to use similar calculations to track progress on their legislative initiatives.
Python libraries make this a breeze, especially when dealing with dates and times across different time zones.
Calculating Script Execution Time
Python’s `time` module provides tools for measuring execution time. A simple example measures the time taken by a loop to process a large dataset.“`pythonimport timeimport randomdef process_data(data): start_time = time.time() for item in data: # Simulate some work random.random() end_time = time.time() return end_time – start_time# Example usagedata = [i for i in range(100000)]execution_time = process_data(data)print(f”Execution time: execution_time:.4f seconds”)“`This code snippet calculates the time difference between the start and end of the `process_data` function, providing a precise measurement of execution time.
This is useful for optimizing algorithms or identifying performance bottlenecks.
Calculating Time Difference from Log Files
Analyzing log files often involves determining the time elapsed between specific events. This example shows how to calculate the time difference between log entries related to file processing.“`pythonimport reimport timedef time_diff_from_log(log_file): time_diffs = [] with open(log_file, ‘r’) as f: lines = f.readlines() for i in range(len(lines)): match = re.search(r”(\d4-\d2-\d2 \d2:\d2:\d2)”, lines[i]) if match: try: start_time = time.mktime(time.strptime(match.group(1), “%Y-%m-%d %H:%M:%S”)) match_next = re.search(r”(\d4-\d2-\d2 \d2:\d2:\d2)”, lines[i+1]) if match_next: end_time = time.mktime(time.strptime(match_next.group(1), “%Y-%m-%d %H:%M:%S”)) time_diff = end_time – start_time time_diffs.append(time_diff) except ValueError: print(f”Error parsing time in line i+1″) continue return time_diffs“`This function parses a log file containing timestamps, identifies relevant lines, and computes the difference between the timestamps, returning a list of time differences.
Calculating Time Difference Between User Logins
Tracking user login times is essential for security and analytics. This example demonstrates how to calculate the time difference between consecutive logins for a user.“`pythonimport pandas as pdimport datetimedef user_login_times(user_login_data): df = pd.DataFrame(user_login_data, columns=[‘user_id’, ‘login_time’]) df[‘login_time’] = pd.to_datetime(df[‘login_time’]) df[‘time_diff’] = df[‘login_time’].diff().dt.total_seconds() return df“`This code snippet uses Pandas to process user login data.
It converts login times to datetime objects, calculates the difference between consecutive logins, and stores the results in a new column.
Time Difference in Data Analysis
Time differences are vital in data analysis. For example, analyzing website traffic, sales data, or user engagement often requires examining the time intervals between events. By calculating time differences, patterns and trends can be identified, leading to better understanding and insights.
Calculating Time Elapsed Between Process Start and End
This example demonstrates calculating the time elapsed between the start and end of a process using the `time` module.“`pythonimport timedef process_time(): start_time = time.time() # Your process here time.sleep(5) end_time = time.time() return end_time – start_timeelapsed_time = process_time()print(f”Process took elapsed_time seconds.”)“`
Calculating time differences in Python is a pretty straightforward task, especially useful for logging events. However, it’s also important to be aware of broader political issues impacting our lives. For example, the recent California Democrats’ decision to delay a vote, to ‘Trump-proof’ the state and protect immigrants, as detailed in this article california democrats delay vote to trump proof the state and protect immigrants , highlights the complex interplay of political and social factors.
Ultimately, mastering time difference calculations in Python is a valuable skill that can be applied to a wide variety of situations, whether personal or professional.
Calculating User Task Time
Calculating the time a user spends on a particular task is valuable for performance analysis or user experience studies. By tracking start and end times, the time spent on specific tasks can be accurately calculated.“`pythonimport datetimedef track_task_time(task_name): start_time = datetime.datetime.now() # Perform the task time.sleep(3) end_time = datetime.datetime.now() task_duration = end_time – start_time print(f”Task ‘task_name’ took task_duration.”)track_task_time(“Data Processing”)“`
Event Time Difference Tracking Program
A comprehensive program tracks and reports time differences between events in a system. The program takes event data, calculates the differences, and presents the results in a user-friendly format, often in a table or graphical representation.
Advanced Techniques
Diving deeper into time manipulation in Python, this section explores advanced techniques for handling various date and time formats, leveraging external libraries, and managing complex time-sensitive operations. These methods are crucial for working with real-world data where dates and times might be presented in diverse formats or require intricate calculations.This section provides practical examples to demonstrate how to parse dates from different formats, format datetime objects, and handle time zones effectively.
Furthermore, we’ll discuss the power of external libraries and regular expressions for extracting dates and times from text, equipping you with robust solutions for your time-related programming tasks.
Parsing Dates and Times with `strptime`
The `datetime.strptime()` method is a powerful tool for converting strings representing dates and times into `datetime` objects. It allows you to parse a wide array of date and time formats.
import datetime date_string = "2024-10-27 10:30:00" date_object = datetime.datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S") print(date_object)
This example demonstrates parsing a date-time string in the format “YYYY-MM-DD HH:MM:SS”. The format string `”%Y-%m-%d %H:%M:%S”` specifies the expected format of the input string. Numerous format codes are available, allowing you to handle various date and time representations.
Formatting `datetime` Objects with `strftime`
Conversely, `datetime.strftime()` allows you to format `datetime` objects into strings in a desired format. This is essential for presenting date and time information in reports, user interfaces, or other applications.
Calculating time differences in Python is a handy skill, especially when dealing with large datasets. For example, imagine tracking the time elapsed between various events, like the surge in Tesla owner protests, which are rippling across the Bay Area , and then comparing that to other potential delays. Understanding time differences is fundamental to analyzing data and finding patterns, a key element in Python programming.
import datetime date_object = datetime.datetime(2024, 10, 27, 10, 30, 0) formatted_string = date_object.strftime("%B %d, %Y") print(formatted_string)
This code converts a `datetime` object into a string formatted as “Month DD, YYYY”.
Handling Different Date Formats
Real-world data often contains dates in diverse formats. You might encounter dates in “DD/MM/YYYY” or “MM/DD/YYYY” formats. Appropriate use of `strptime` with tailored format codes is crucial to handle these scenarios.
import datetime date_string_1 = "27/10/2024" date_object_1 = datetime.datetime.strptime(date_string_1, "%d/%m/%Y") print(date_object_1) date_string_2 = "10/27/2024" date_object_2 = datetime.datetime.strptime(date_string_2, "%m/%d/%Y") print(date_object_2)
These examples demonstrate handling dates in “DD/MM/YYYY” and “MM/DD/YYYY” formats, respectively. Adjusting the format code in `strptime` is essential to correctly interpret the input string.
Beyond Standard Modules
Libraries like `pendulum` provide additional features for timezone handling, parsing various date/time formats, and calculations. These libraries enhance the capabilities of Python’s built-in `datetime` module.
Advanced Time Calculations
Libraries like `arrow` or `dateutil` offer more sophisticated time calculations, including handling time zones and complex date manipulations.
Regular Expressions for Date Extraction
Regular expressions can be used to extract dates and times from text. This is particularly useful when dealing with unstructured data or log files.
import re text = "The meeting is scheduled for October 27, 2024 at 10:30 AM." match = re.search(r"(\w+ \d+, \d+)", text) if match: date_extracted = match.group(1) print(date_extracted)
This example extracts the date from the text using a regular expression.
Best Practices and Error Handling
Calculating time differences accurately and reliably in Python requires careful consideration of potential pitfalls and robust error handling. This section delves into best practices for avoiding common errors and ensuring the integrity of your time calculations. Proper error handling not only prevents unexpected crashes but also provides informative feedback to the user, improving the overall usability of your application.
Best Practices for Time Difference Calculations
Robust time difference calculations necessitate adherence to best practices. Using appropriate Python libraries, meticulously validating input data, and implementing clear error handling mechanisms are crucial. Employing consistent units for time intervals, and avoiding implicit type conversions, are key elements of ensuring accurate results.
Handling Invalid Date Formats
Incorrectly formatted dates can lead to unpredictable results or program crashes. Input validation is essential to prevent these issues. Employing functions to parse dates in a controlled manner and raise exceptions for invalid formats is critical. This approach ensures data integrity and prevents silent errors that can be difficult to track down.
Handling Time Zone Issues
Time zone inconsistencies can easily introduce errors in time difference calculations. Explicitly handling time zones using the `datetime` module or dedicated libraries is crucial. Convert all timestamps to a common time zone before performing calculations to prevent discrepancies arising from different time zones. This ensures consistent and accurate time comparisons.
Ensuring Accuracy and Reliability
Ensuring accuracy and reliability in time calculations involves careful consideration of various factors. Using appropriate data types and adhering to best practices for time zone handling are vital. Employing consistent units for time intervals helps avoid confusion and ensure correct results. Testing your calculations with various input data, including edge cases, helps identify potential problems and improve the robustness of your code.
Common Pitfalls to Avoid
Several common pitfalls can lead to errors in time difference calculations. Implicit type conversions can lead to unexpected results, while neglecting to handle time zone information can introduce discrepancies. Improperly formatted input data can lead to crashes or incorrect output. Using incorrect units for time intervals can obscure the meaning of the results.
Error Handling Strategies
Effective error handling is crucial to maintaining the stability and reliability of your code. Implementing `try…except` blocks can catch potential errors, such as `ValueError` for invalid dates or `TypeError` for incompatible data types. Using informative error messages helps in debugging and maintaining the code.
Documenting and Maintaining Time Calculation Code
Proper documentation and maintenance are essential for time calculation code. Clear variable names, well-commented code, and detailed explanations of the calculation logic enhance readability and maintainability. Using a consistent style guide ensures that the code is easy to understand and modify. Regular code reviews can help identify potential issues and improve the overall quality of the code.
Common Error Scenarios and Resolutions
Error Scenario | Resolution |
---|---|
Incorrect date format | Use `strptime` to parse dates in a controlled manner, raising `ValueError` for invalid formats. |
Ignoring time zones | Convert all timestamps to a common time zone before performing calculations. |
Implicit type conversions | Explicitly convert data types to the appropriate formats (e.g., `datetime` objects) before performing calculations. |
Incorrect units | Ensure consistent units for time intervals (e.g., seconds, minutes, hours). |
Summary
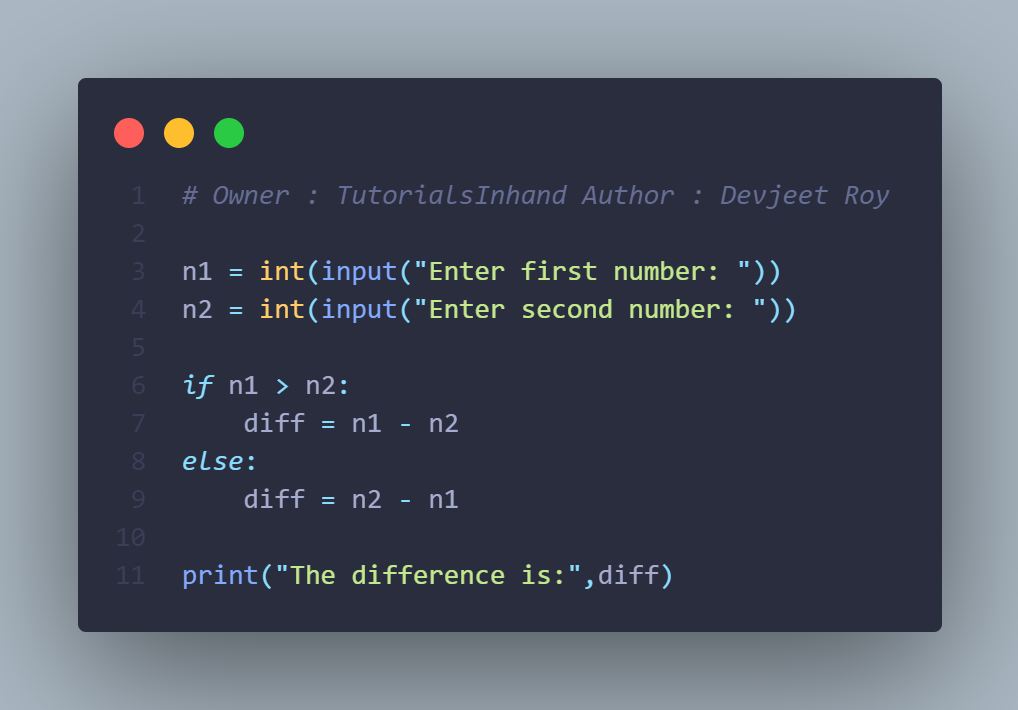
In conclusion, calculating time differences in Python is a valuable skill applicable to a broad spectrum of tasks. By mastering the concepts and techniques discussed here, you can confidently and accurately measure time intervals, ensuring your programs are reliable and efficient. Remember to consider time zones and handle potential errors for optimal results. Happy coding!