Create a Blockchain with Python A Deep Dive
Create a blockchain with Python sets the stage for this enthralling narrative, offering readers a glimpse into the intricate world of distributed ledger technology. We’ll explore the fundamental concepts of blockchain, from its core principles to various architectures, and delve into the practical aspects of implementing a blockchain using Python. This journey will cover everything from setting up the basic structure to understanding advanced features like smart contracts and consensus mechanisms.
This comprehensive guide will equip you with the knowledge and tools to build your own blockchain application using Python, demonstrating the power and versatility of this popular language in the realm of decentralized technologies. We’ll walk through step-by-step instructions, practical code examples, and essential considerations for creating a robust and secure blockchain.
Introduction to Blockchain
Blockchain technology is a revolutionary approach to data management, characterized by its decentralized and immutable nature. It essentially creates a shared, distributed ledger that records transactions across multiple computers. This shared record is secure and transparent, making it a powerful tool for various applications, from cryptocurrency to supply chain management. The core principles of blockchain are immutability, transparency, and decentralization.Blockchain technology, in its essence, functions as a continuously growing list of records, called blocks.
Each block is linked to the previous one, forming a chain. This linked structure, combined with cryptographic hashing, ensures that once a block is added to the chain, it cannot be altered or deleted. This fundamental feature of immutability is what makes blockchain so attractive for applications requiring trust and transparency.
Types of Blockchains
Different blockchain architectures cater to various needs and applications. Understanding these distinctions is crucial to appreciating the versatility of blockchain technology.
- Public Blockchains:
- Public blockchains, like Bitcoin, are open to anyone. Anyone can participate in the network, contribute to the ledger, and verify transactions. This inherent openness fosters transparency and trust. The lack of central control is a defining characteristic of public blockchains.
- Private Blockchains:
- In contrast to public blockchains, private blockchains are permissioned. Access to the network and participation in the ledger are controlled by a designated group. This control is essential for industries where strict confidentiality and security are paramount. The focus on control and privacy makes private blockchains ideal for specific use cases.
- Consortium Blockchains:
- Consortium blockchains represent a middle ground. These networks are permissioned, but participation is limited to a specific group of pre-approved entities, often representing different organizations within an industry. This allows for controlled access while maintaining a level of decentralization, often preferred in situations where multiple parties need to share data in a controlled and transparent manner.
Blockchain Architectures
The architectural approach to a blockchain system significantly impacts its functionality and suitability.
- Permissioned Blockchains:
- Permissioned blockchains, as the name implies, require prior authorization for participation. This approach, common in private and consortium blockchains, prioritizes control and security. Examples include supply chain management systems where specific entities need to contribute to and access the ledger.
- Permissionless Blockchains:
- Permissionless blockchains, such as Bitcoin, do not require any pre-approval. This characteristic allows for broad participation and transparency. Anyone can join the network, participate in transaction verification, and contribute to the ledger, which is a core tenet of decentralization. The absence of pre-approval makes them suitable for applications where broad participation and transparency are critical.
Cryptography in Blockchain Security
Cryptography plays a vital role in securing blockchain systems. It underpins the entire architecture, ensuring the integrity and immutability of the data.
Cryptographic hashing algorithms generate unique fingerprints (hashes) for each block. These hashes are crucial for verifying the integrity of the data and ensuring that no block has been tampered with.
Cryptography’s role in blockchain security extends to digital signatures, ensuring the authenticity of transactions and the identity of participants. This robust system of security mechanisms creates a reliable and trustworthy environment for various applications.
Comparison of Public and Private Blockchains
Feature | Public Blockchain | Private Blockchain |
---|---|---|
Access | Open to anyone | Restricted to authorized participants |
Control | Decentralized | Centralized or semi-centralized |
Transparency | High | Low to moderate |
Security | High, due to distributed nature | High, due to controlled access |
Scalability | Potentially lower | Potentially higher |
Use Cases | Cryptocurrencies, decentralized applications (dApps) | Supply chain management, internal record keeping, financial transactions within an organization |
Python for Blockchain Development
Python’s versatility and extensive libraries make it a compelling choice for blockchain development. Its readability and ease of use contribute to faster development cycles, allowing developers to focus on the core logic rather than complex low-level details. This is particularly valuable when prototyping or building smaller-scale blockchain applications.Python’s rich ecosystem of libraries offers a variety of tools tailored for different stages of blockchain development.
This, combined with Python’s active community and readily available resources, makes it an attractive option for beginners and experienced blockchain developers alike. However, it’s important to understand Python’s limitations when dealing with high-performance and computationally intensive tasks.
Popular Python Libraries for Blockchain
Python offers a range of libraries that facilitate blockchain development. Key libraries include those supporting cryptography, data structures, and network communication. Understanding these libraries empowers developers to create robust and secure blockchain implementations.
- Cryptography Libraries (e.g., cryptography): These libraries provide secure cryptographic functions crucial for blockchain security. They handle tasks like hashing, digital signatures, and encryption, which are fundamental for ensuring data integrity and authenticity. This is critical for protecting transactions and maintaining the ledger’s integrity. The cryptography library is a popular choice due to its comprehensive suite of cryptographic primitives.
- Data Structures and Manipulation (e.g., Pandas, NumPy): Data structures are fundamental to organizing and managing blockchain data. Libraries like Pandas and NumPy are invaluable for working with large datasets, enabling efficient storage and retrieval of transaction information. NumPy, for instance, offers optimized numerical computations. This is vital for handling potentially enormous amounts of data.
- Networking Libraries (e.g., asyncio, Socket): Blockchain networks require robust communication mechanisms. Python’s networking libraries, such as asyncio and Socket, are critical for enabling nodes to communicate and validate transactions efficiently. These libraries ensure smooth data exchange among nodes in a blockchain network.
Advantages of Using Python for Blockchain
Python’s advantages in blockchain development stem from its strengths as a programming language. Its readability, extensive libraries, and active community significantly accelerate development cycles.
- Readability and Ease of Use: Python’s clear syntax and extensive libraries make it user-friendly for developers, particularly those new to blockchain. This simplifies the development process, especially for projects with tight deadlines or those involving multiple developers.
- Extensive Libraries: The rich ecosystem of Python libraries provides ready-made solutions for common tasks in blockchain development, including cryptography, data structures, and networking. This reduces the need to write custom code, accelerating development and lowering the risk of errors.
- Active Community and Resources: A large and active community provides ample support and readily available resources, such as tutorials, documentation, and forums. This assistance proves beneficial for developers encountering challenges or seeking best practices.
Disadvantages of Using Python for Blockchain
Despite its advantages, Python faces limitations in blockchain development, particularly concerning performance.
Learning to create a blockchain with Python is fascinating, especially when you consider real-world applications like ensuring fair elections. For example, the recent Gilroy voting rights election change districts decision lawsuit here highlights the importance of transparent and secure systems. This underscores the need for blockchain technology to potentially safeguard future elections. Ultimately, building a robust blockchain with Python offers solutions to many challenges, including those related to fair and transparent elections.
- Performance Considerations: Python’s interpreted nature can lead to slower execution speeds compared to compiled languages like C++ or Java. This can be a concern for high-performance applications or large-scale blockchain networks.
- Scalability Challenges: In certain scenarios, Python’s performance limitations can pose challenges in handling a high volume of transactions. This issue is critical for blockchain networks designed to process numerous transactions concurrently.
Comparison with Other Languages
Python’s suitability for blockchain development depends on the specific project requirements. A comparative analysis with other languages helps in making informed decisions.
Language | Strengths | Weaknesses |
---|---|---|
Python | Readability, ease of use, extensive libraries | Performance limitations, scalability challenges |
C++ | High performance, low-level control | Steeper learning curve, less readable |
Java | Robustness, platform independence | Can be verbose, potentially slower |
Essential Data Structures and Algorithms
The design of a blockchain requires specific data structures and algorithms for efficiency and security.
- Block Structure: A block typically includes a timestamp, a hash of the previous block, a list of transactions, and a hash of the data itself. This structure ensures the integrity and chronological order of the blockchain.
- Hashing Algorithms: Cryptographic hashing functions are essential for securing the blockchain. They generate unique fingerprints for blocks, ensuring any changes are immediately detectable.
- Consensus Mechanisms: Mechanisms like Proof-of-Work or Proof-of-Stake are critical for ensuring agreement among network nodes regarding the state of the blockchain. This maintains consistency and prevents fraudulent activity.
Creating a Basic Blockchain Structure in Python
Implementing a basic blockchain structure involves several key steps, highlighting the core components of the blockchain.
- Define the Block Structure: A class or dictionary represents a block, encapsulating the data. The structure should include fields for transaction data, the hash of the previous block, a timestamp, and a hash of the block itself.
- Implement the Hashing Function: A function computes the cryptographic hash of the block, which is crucial for integrity verification. Secure hashing functions are fundamental to maintaining the integrity of the blockchain.
- Create the Blockchain Class: The class manages the chain of blocks, including adding new blocks, validating transactions, and maintaining the chain’s integrity. The class is responsible for managing the block structure and the overall blockchain.
Implementing a Simple Blockchain in Python
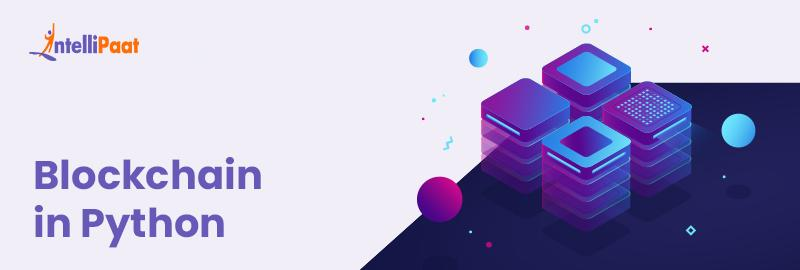
Building a blockchain from scratch is a rewarding exercise. It allows us to understand the core principles of decentralization, immutability, and transparency. This exploration delves into the practical implementation of a basic blockchain using Python, demonstrating the crucial elements of block creation, chain validation, and cryptographic security.This hands-on approach will walk you through the steps involved in building a rudimentary blockchain system.
We’ll use Python’s powerful libraries to implement cryptographic hashing and digital signatures, essential for maintaining the integrity of the blockchain. This will provide a foundation for understanding more complex blockchain applications.
Basic Blockchain Structure
A blockchain is a chain of blocks, each containing a set of transactions. Crucially, each block contains a cryptographic hash of the previous block, forming a linked list. This crucial link ensures the integrity of the entire chain. A block’s immutability comes from this chain of linked hashes. If any data within a block is tampered with, its hash will change, and the following blocks in the chain will be invalidated.
Block Structure
The structure of a blockchain block is fundamental to its operation. A block typically contains several key fields:
Field | Description |
---|---|
Timestamp | The time when the block was created. |
Data | The set of transactions within the block. |
Previous Hash | The cryptographic hash of the preceding block in the chain. This is the crucial link that establishes the chain’s immutability. |
Hash | The cryptographic hash of the block’s contents (timestamp, data, previous hash). This ensures data integrity. |
Block Creation
The process of creating a new block involves several steps. First, a timestamp is recorded. Next, the data for the block is collected. This data could be transactions or any other relevant information. Subsequently, the hash of the previous block is retrieved.
The data, timestamp, and previous hash are then combined, and a cryptographic hash function is applied to generate the current block’s hash.
Chain Validation
Validating a blockchain involves ensuring that each block’s hash matches the expected value based on its contents and the hash of the preceding block. This step is crucial to identify any tampering that may have occurred. If a block’s hash does not match, the chain is deemed invalid.
Python Implementation
This section Artikels the Python implementation for a basic blockchain. We’ll use the `hashlib` library for cryptographic hashing and the `datetime` module for timestamps.“`pythonimport hashlibimport datetimeclass Block: def __init__(self, timestamp, data, previous_hash): self.timestamp = timestamp self.data = data self.previous_hash = previous_hash self.hash = self.calculate_hash() def calculate_hash(self): # …
(hash calculation using hashlib) … return hashlib.sha256(str(self.timestamp + self.data + self.previous_hash).encode()).hexdigest()# Example usage (for demonstration purposes only):genesis_block = Block(datetime.datetime.now(), “Genesis Block”, “0”)“`This is a simplified example. A real-world implementation would include more robust error handling, security measures, and optimized hashing algorithms.
Key Concepts and Considerations
Building a blockchain involves more than just code; it necessitates a deep understanding of fundamental concepts and potential pitfalls. This section delves into crucial aspects of distributed ledger technology, decentralization challenges, scalability issues, and security vulnerabilities, providing a more nuanced perspective for aspiring blockchain developers.
Distributed Ledger Technology (DLT) and Blockchain
Distributed Ledger Technology (DLT) is a shared, replicated database managed across multiple computers. A blockchain is a specific type of DLT, characterized by its immutability and cryptographic security. The distributed nature of a blockchain ensures data integrity and transparency by eliminating a single point of failure. This shared ledger fosters trust among participants, reducing the need for intermediaries.
The immutability of the chain is crucial in maintaining data consistency and preventing tampering.
Challenges of Implementing a Decentralized System in Python
Developing a decentralized system in Python presents unique challenges. One key difficulty lies in ensuring the integrity and consistency of the distributed ledger across multiple nodes. Maintaining consensus among nodes, especially in a large network, requires robust mechanisms and efficient communication protocols. Ensuring data integrity across multiple nodes in a distributed system demands strong cryptographic techniques and secure data structures.
Ever wanted to dive into creating a blockchain with Python? It’s a fascinating project, and you could potentially use it for some pretty cool applications, like streamlining the logistics of climate clean American ports. Climate clean American ports are a hot topic, and a blockchain could really improve transparency and efficiency in supply chains. Thinking about how Python could be used to build this kind of blockchain is incredibly interesting.
Achieving true decentralization, where no single entity controls the system, can be complex.
Scalability and Performance Challenges in Blockchain Development
Scalability and performance are critical considerations in blockchain development. As the network grows, transaction throughput needs to increase to avoid bottlenecks. The time it takes to add new blocks can impact the user experience and overall network performance. The block size limitation in many blockchain implementations restricts the number of transactions that can be processed in a single block, potentially causing delays.
Scalability solutions, such as sharding or layer-2 solutions, are often employed to address these issues. For instance, Bitcoin’s scalability limitations have spurred exploration of alternative blockchain architectures.
Potential Security Vulnerabilities in Blockchain Implementation
Security is paramount in blockchain development. Common vulnerabilities include vulnerabilities in the cryptographic algorithms used to secure transactions, and the potential for attacks like 51% attacks, which occur when a malicious actor controls more than half of the network’s computational power. Smart contract vulnerabilities, crucial for blockchain applications, are a critical area of concern. Implementing robust security measures and rigorous code reviews are essential to mitigate these risks.
Also, issues with node security and the potential for denial-of-service attacks are crucial to address.
Summary of Common Challenges in Python Blockchain Development
Challenge Category | Specific Challenges |
---|---|
Decentralization | Maintaining consensus among nodes, ensuring data integrity, achieving true decentralization. |
Scalability | Transaction throughput, block time, block size limitations. |
Security | Cryptographic vulnerabilities, 51% attacks, smart contract vulnerabilities, node security, denial-of-service attacks. |
Performance | Transaction processing speed, network latency, data storage efficiency. |
Advanced Blockchain Features (Optional): Create A Blockchain With Python
Building upon a basic blockchain, incorporating advanced features like smart contracts, diverse consensus mechanisms, and cryptocurrency integration elevates its capabilities. These enhancements enable more complex applications, from decentralized finance to secure voting systems. The flexibility and security of a robust blockchain underpin these advanced applications.
Smart Contract Integration
Smart contracts automate agreements by codifying predefined rules directly onto the blockchain. These self-executing contracts facilitate trustless transactions and eliminate the need for intermediaries. Integrating smart contracts into a Python blockchain involves defining the contract logic using a suitable language like Solidity or Vyper. This logic is then deployed to the blockchain and executed automatically based on predetermined conditions.
The Python blockchain interacts with this deployed contract, ensuring the integrity of the transaction.
Consensus Mechanism Implementation
Different consensus mechanisms ensure agreement on the blockchain’s state. Proof-of-Work (PoW) and Proof-of-Stake (PoS) are prominent examples. PoW relies on computational power to validate transactions, while PoS uses the stake held by participants. Implementing PoW involves complex cryptographic hashing algorithms. PoS, on the other hand, leverages the stake of participants in the network, which can be implemented through a modified blockchain consensus protocol.
The choice of consensus mechanism depends on the specific needs and priorities of the blockchain.
Cryptocurrency Transaction Integration
Cryptocurrency transactions are core to blockchain functionality. Implementing cryptocurrency transactions requires creating a system for managing tokens, tracking balances, and facilitating transfers. This involves implementing the appropriate data structures and algorithms for representing and manipulating these tokens. The transaction process includes generating transaction hashes, verifying signatures, and updating balances across the network.
Wallet and Account Management
Managing wallets and accounts is crucial for user interaction with the blockchain. A robust wallet system allows users to securely store and manage their cryptocurrencies. The implementation entails creating methods for generating and managing private and public keys, handling cryptographic operations, and enabling secure storage of sensitive information. This includes functionalities for sending, receiving, and viewing transaction histories.
Consensus Mechanism Comparison
Consensus Mechanism | Description | Characteristics |
---|---|---|
Proof-of-Work (PoW) | Transactions are verified by solving computationally intensive puzzles. | High security, but resource-intensive and energy-consuming. |
Proof-of-Stake (PoS) | Transactions are verified by participants who have staked a certain amount of cryptocurrency. | More energy-efficient than PoW, but potentially susceptible to attacks if validators are not sufficiently incentivized. |
Delegated Proof-of-Stake (DPoS) | A subset of validators is chosen to verify transactions, typically based on their reputation or stake. | Improved scalability compared to PoS, but can potentially lead to centralization. |
Real-World Applications
Blockchain technology, once relegated to the realm of cryptocurrencies, is rapidly expanding its influence across various industries. Its inherent security, transparency, and immutability offer significant advantages over traditional systems, driving innovation and efficiency in diverse sectors. This section explores practical applications, potential impacts, and the challenges that remain in the adoption of blockchain technology.The potential of blockchain extends beyond digital currencies.
Creating a blockchain with Python can be a fascinating project, but it’s also important to consider how AI can enhance its applications. Thinking about the best AI agents for business, like those found at best ai agents for business , could significantly boost the blockchain’s capabilities. Ultimately, integrating smart contracts with cutting-edge AI solutions will be crucial for the future of blockchain technology.
Its core principles of decentralization and secure record-keeping make it a compelling solution for streamlining processes and enhancing trust in a wide range of transactions and data management systems. From supply chain management to voting systems, blockchain has the potential to revolutionize how we conduct business and interact with institutions.
Blockchain Applications in Diverse Industries
Blockchain’s versatility is evident in its adoption across various sectors. Its ability to create tamper-proof records is proving invaluable in situations where trust and transparency are paramount.
- Supply Chain Management: Blockchain allows for complete traceability of goods throughout the supply chain, from origin to consumer. This enhanced transparency can help track products, identify counterfeits, and improve ethical sourcing. For instance, companies like Walmart and IBM have been experimenting with blockchain-based supply chain solutions to ensure product authenticity and improve the efficiency of their logistics networks.
- Healthcare: Blockchain can securely store and share patient medical records, enhancing data privacy and facilitating secure access for authorized individuals. This has the potential to revolutionize how medical information is managed and shared, particularly in international contexts where cross-border data transfer poses challenges.
- Finance: Cryptocurrencies like Bitcoin and Ethereum rely on blockchain technology. Beyond cryptocurrencies, blockchain is being explored for faster and cheaper cross-border payments, streamlining financial transactions, and reducing fraud.
- Voting Systems: Blockchain’s inherent security and transparency could potentially create more secure and auditable voting systems, mitigating the risk of fraud and enhancing public trust in electoral processes. This is particularly relevant in regions with a history of election-related concerns.
Potential Impact on Different Sectors
Blockchain’s transformative potential extends to many industries, impacting how businesses operate and how people interact with institutions.
- Increased Transparency and Trust: The immutable nature of blockchain fosters greater trust between parties involved in transactions, especially in areas like supply chain management and healthcare. This increased transparency can lead to reduced fraud and improved efficiency.
- Improved Efficiency and Reduced Costs: Automation of processes through blockchain can reduce paperwork, streamline operations, and decrease transaction costs. This is particularly beneficial in supply chain management and financial transactions.
- Enhanced Security and Data Integrity: The decentralized nature of blockchain makes it more resistant to data breaches and tampering, which is critical in industries like healthcare and finance where data security is paramount.
Current Limitations and Future Prospects
Despite its potential, blockchain technology faces some limitations. Scalability remains a significant challenge, particularly for high-volume transactions. Regulatory uncertainties also hinder widespread adoption. However, ongoing research and development are addressing these issues.
- Scalability Issues: Some blockchain networks struggle to handle a large volume of transactions efficiently, potentially slowing down processes in high-transaction industries like finance.
- Regulatory Uncertainty: Lack of clear regulatory frameworks in many jurisdictions can deter businesses from adopting blockchain solutions, especially when it comes to compliance.
- High Energy Consumption: Some blockchain technologies, particularly those using Proof-of-Work consensus mechanisms, can consume significant energy resources. Efforts to develop more energy-efficient consensus mechanisms are underway.
Table of Diverse Use Cases
The following table highlights various applications of blockchain technology across different sectors.
Sector | Use Case |
---|---|
Supply Chain | Tracking goods, verifying authenticity, improving transparency |
Healthcare | Secure storage and sharing of patient records, improving data privacy |
Finance | Faster and cheaper cross-border payments, reducing fraud |
Voting | Creating more secure and auditable voting systems |
Real Estate | Registering and transferring property ownership securely |
Implications of Blockchain Adoption in Supply Chain Management
Blockchain’s impact on supply chain management is profound, transforming how goods are tracked and managed.
- Enhanced Transparency: Every stage of the supply chain, from sourcing to delivery, can be tracked on a shared, immutable ledger. This provides greater visibility and accountability.
- Reduced Fraud and Counterfeiting: Tamper-proof records make it easier to identify and prevent fraudulent activities and counterfeit products.
- Improved Efficiency and Cost Savings: Streamlined processes and reduced paperwork contribute to increased efficiency and lower costs.
- Ethical Sourcing: Blockchain can help companies ensure ethical and sustainable sourcing practices throughout their supply chain.
Example Code Snippets
Diving deeper into the practical side of blockchain development, this section provides concise code snippets illustrating essential blockchain operations. We’ll explore how to create blocks, add transactions, validate the chain, implement hashing algorithms, and handle cryptographic keys in Python. These examples provide a foundation for building more complex and robust blockchain applications.
Creating a Block
Creating a block involves packaging data with cryptographic hashes. The code below demonstrates a basic block structure, including the timestamp, previous hash, and data. A crucial element is the use of a hashing algorithm (SHA-256 in this case) to secure the block’s integrity.
“`pythonimport hashlibimport datetimeclass Block: def __init__(self, timestamp, previous_hash, data): self.timestamp = timestamp self.previous_hash = previous_hash self.data = data self.hash = self.calculate_hash() def calculate_hash(self): sha = hashlib.sha256() block_string = str(self.timestamp) + str(self.previous_hash) + str(self.data) sha.update(block_string.encode(‘utf-8’)) return sha.hexdigest()“`
Adding a Transaction, Create a blockchain with python
Adding a transaction to the blockchain involves appending a transaction record to a block. This example demonstrates how a transaction record can be added to the block, with the timestamp and transaction details included.
“`python# Example of adding a transactionnew_transaction = ‘sender’: ‘Alice’, ‘receiver’: ‘Bob’, ‘amount’: 10“`
Validating the Blockchain
Validating the blockchain ensures the integrity of the chain by verifying that each block’s hash is correct. The code below showcases the validation process, comparing the calculated hash with the stored hash.
“`pythondef is_valid_chain(chain): for i in range(1, len(chain)): current_block = chain[i] previous_block = chain[i-1] if current_block.hash != current_block.calculate_hash(): return False if current_block.previous_hash != previous_block.hash: return False return True“`
Implementing Hashing Algorithms
Hashing algorithms are fundamental for blockchain security. The code snippet demonstrates how to use the SHA-256 hashing algorithm, a widely used cryptographic hash function.
“`pythonimport hashlibdef hash_string(string): sha = hashlib.sha256() sha.update(string.encode(‘utf-8’)) return sha.hexdigest()“`
Handling Cryptographic Keys
Cryptographic keys are crucial for securing transactions and validating identities. This example demonstrates how to generate and use public and private keys, using a suitable cryptography library. For security, always use strong, randomly generated keys.
“`pythonfrom cryptography.hazmat.primitives.asymmetric import rsafrom cryptography.hazmat.backends import default_backenddef generate_keys(): private_key = rsa.generate_private_key( public_exponent=65537, key_size=2048, backend=default_backend() ) public_key = private_key.public_key() return private_key, public_key“`
Closing Notes
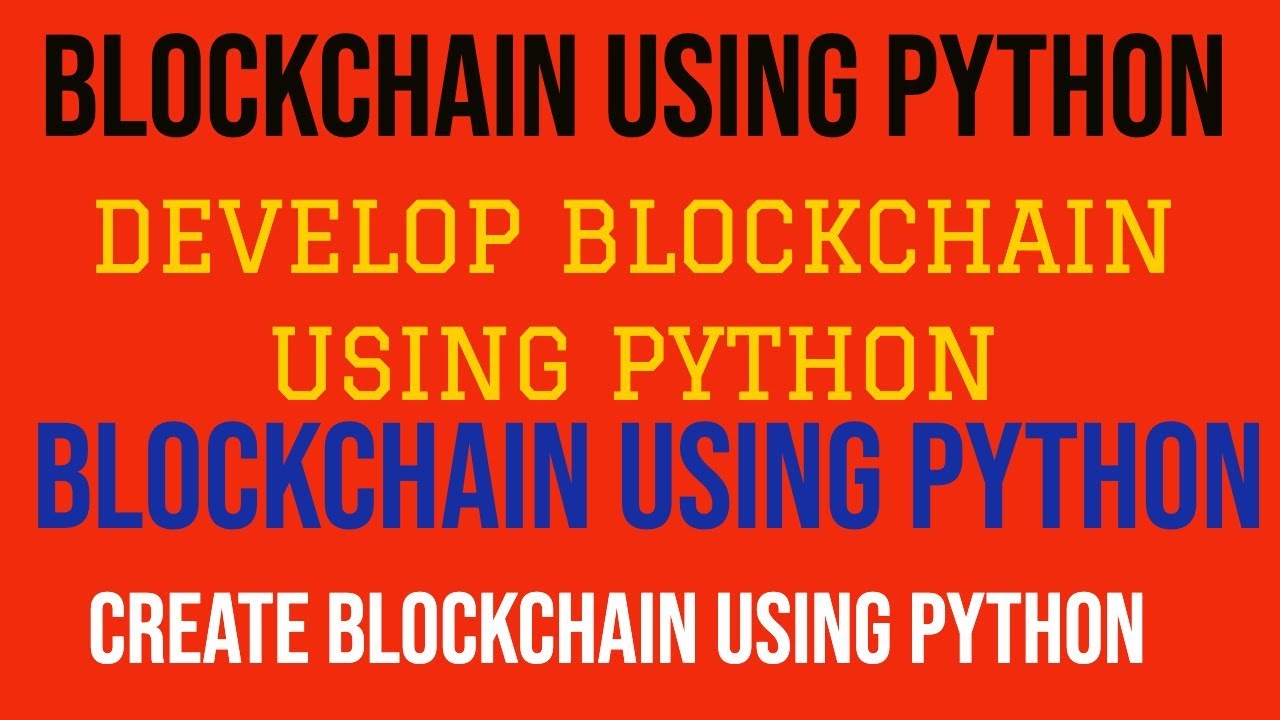
In conclusion, creating a blockchain with Python is a fascinating journey into the world of decentralized applications. We’ve covered the fundamental building blocks, practical implementation strategies, and key considerations. From the basics of blockchain technology to the practical implementation using Python, this guide provides a strong foundation for understanding and applying blockchain principles. We encourage you to experiment with the code snippets and explore the numerous possibilities for blockchain applications in various sectors.