Enable CORS Apache Nginx A Comprehensive Guide
Enable CORS Apache Nginx is crucial for modern web applications, especially when front-end interfaces need to communicate with back-end APIs hosted on different servers. This guide delves into the intricacies of configuring CORS support on both Apache and Nginx servers, covering everything from fundamental concepts to advanced configurations and security considerations. We’ll explore the necessary steps to set up CORS, troubleshoot common errors, and understand its impact on application performance.
Understanding CORS is essential for seamless communication between different domains. This guide walks through the configuration process, providing practical examples and highlighting best practices for both Apache and Nginx. From basic setup to advanced scenarios, we’ll cover all the key aspects of implementing CORS effectively.
Introduction to CORS
Cross-Origin Resource Sharing (CORS) is a mechanism that allows restricted resources on a server to be accessed from a different domain. This is crucial for enabling communication between different websites or web applications, especially in scenarios where a client-side application needs to fetch data from a server on a different domain. Without CORS, browsers impose security restrictions to prevent malicious scripts from accessing resources from other domains.CORS fundamentally addresses the same-origin policy enforced by web browsers.
This policy restricts a script from one origin (domain, protocol, and port) from accessing resources from a different origin. This policy is a crucial security measure to protect users from malicious websites trying to steal data or inject scripts into a legitimate website. CORS provides a controlled mechanism to relax these restrictions in specific situations where it’s safe and beneficial for the user.
CORS Functionality
CORS operates by allowing the server to explicitly specify which origins are allowed to access its resources. The server sends headers to the browser, detailing which origins are permitted. The browser then uses these headers to determine whether or not to allow the request to proceed. This allows the server to define the specific domains and types of requests that can access the resources.
Typical Use Cases
CORS is essential in various web application scenarios. One prominent use case is single-page applications (SPAs) that frequently fetch data from APIs hosted on different domains. Another use case involves third-party integrations where a website needs to access data from an external service. Furthermore, CORS is crucial for web services and APIs, enabling client-side applications to retrieve data from a remote server without security concerns.
A prime example is a social media platform where users might access data from other applications, such as news feeds or photo sharing sites.
Client-Server Interaction Example
The following table demonstrates a simple client-server interaction requiring CORS:
Client Origin | Server Origin | Resource | Request Type |
---|---|---|---|
https://client.example.com |
https://api.example.com |
/data |
GET |
In this example, the client, hosted on https://client.example.com
, needs to retrieve data from the server https://api.example.com
. Due to the different origins, the browser will block the request without CORS configuration on the server. The server, using CORS headers, would explicitly allow the client to access the resource, thereby enabling the client to fetch data from the API.
CORS Implementation with Apache: Enable Cors Apache Nginx
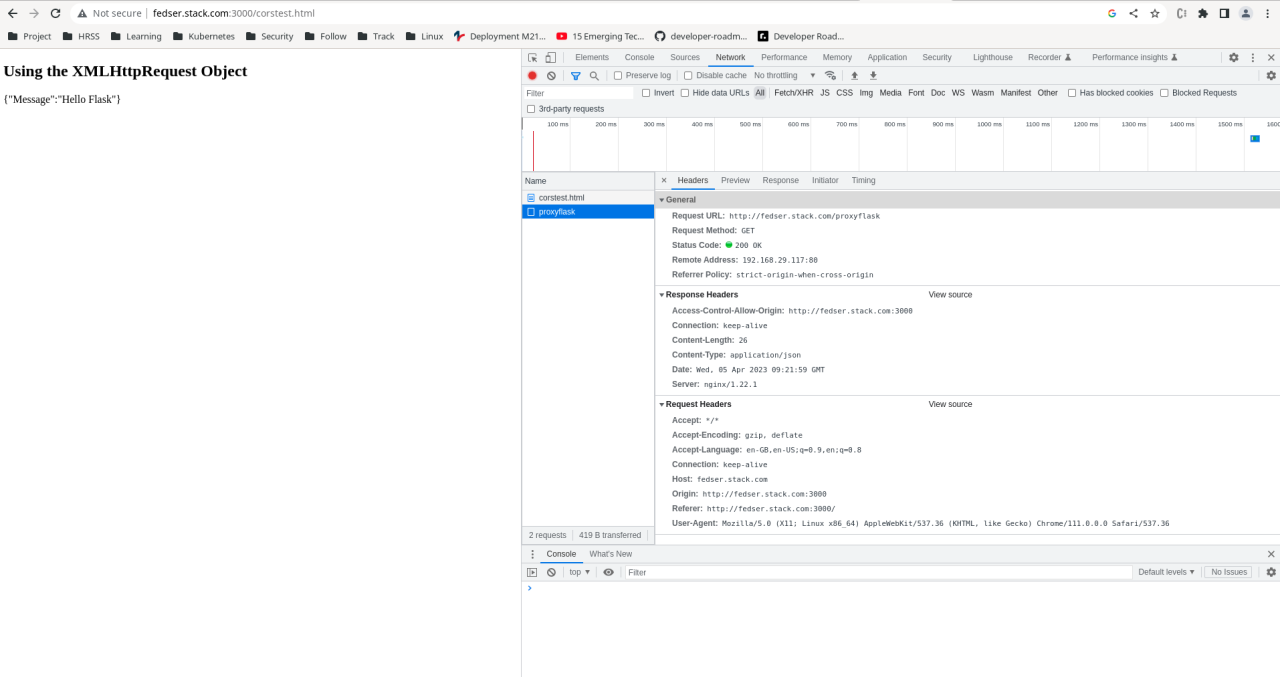
Apache HTTP Server provides a robust mechanism for enabling Cross-Origin Resource Sharing (CORS). This involves configuring the server to respond to CORS preflight requests and allow specific origins, methods, and headers. This allows web applications hosted on different domains to interact securely with resources on the Apache server.Understanding CORS is crucial for modern web development. Without proper CORS configuration, your web application might encounter errors when trying to access resources from different domains.
This is where Apache’s configuration directives come into play.
Configuring CORS in Apache
The core of enabling CORS in Apache lies in strategically using HTTP headers in response to preflight requests. These headers inform the client about the permissible origins, methods, and headers for subsequent requests. Apache’s mod_headers module is essential for this process.
Apache Configuration Directives
Configuring CORS in Apache involves several directives within the `httpd.conf` or `.htaccess` file. These directives define the rules for handling CORS requests. Crucial directives include:
Header set Access-Control-Allow-Origin "your-origin"
: This directive sets the `Access-Control-Allow-Origin` header, specifying the allowed origin for requests. Replace `”your-origin”` with the actual origin (e.g., `https://example.com`). This is the fundamental directive for CORS.Header set Access-Control-Allow-Methods "GET, POST, PUT, DELETE"
: Defines the HTTP methods allowed for cross-origin requests. Replace the example methods with the appropriate ones (e.g., `GET`, `POST`, `OPTIONS`, etc.).Header set Access-Control-Allow-Headers "Content-Type, Authorization"
: Specifies the headers allowed in the request. This ensures that the server accepts specific headers for requests. Adjust this list to match your application’s needs.Header set Access-Control-Max-Age "86400"
: Specifies the duration (in seconds) for which the preflight response is valid. A longer value reduces the frequency of preflight requests.Header always append Access-Control-Allow-Credentials "true"
: If your application needs to send cookies with requests, this is essential. It allows the browser to send credentials (like cookies) along with the request.
Example Apache Configuration Snippets
Different CORS scenarios require tailored configurations. Here are examples:
- Allowing requests from a single origin:
Header set Access-Control-Allow-Origin "https://example.com" Header set Access-Control-Allow-Methods "GET, POST" Header set Access-Control-Allow-Headers "Content-Type"
This example allows only requests from `https://example.com` using `GET` and `POST` methods, with `Content-Type` header.
- Allowing requests from multiple origins:
Header set Access-Control-Allow-Origin "https://example.com, https://another-example.com" Header set Access-Control-Allow-Methods "GET, POST, OPTIONS" Header set Access-Control-Allow-Headers "Content-Type, X-Custom-Header"
This snippet allows requests from `https://example.com` and `https://another-example.com`, using `GET`, `POST`, and `OPTIONS` methods, with `Content-Type` and `X-Custom-Header` headers.
Table of CORS Configurations
This table demonstrates variations in configurations based on differing CORS requirements:
Scenario | Access-Control-Allow-Origin | Access-Control-Allow-Methods | Access-Control-Allow-Headers | |
---|---|---|---|---|
Single Origin, Basic Methods | `https://example.com` | `GET, POST` | `Content-Type` | |
Multiple Origins, Extended Methods | `https://example.com, https://another.com` | `GET, POST, PUT, DELETE, OPTIONS` | `Content-Type, Authorization` | |
Credentials Allowed | `https://example.com` | `GET, POST` | `Content-Type` | `Header always append Access-Control-Allow-Credentials “true”` |
CORS Implementation with Nginx
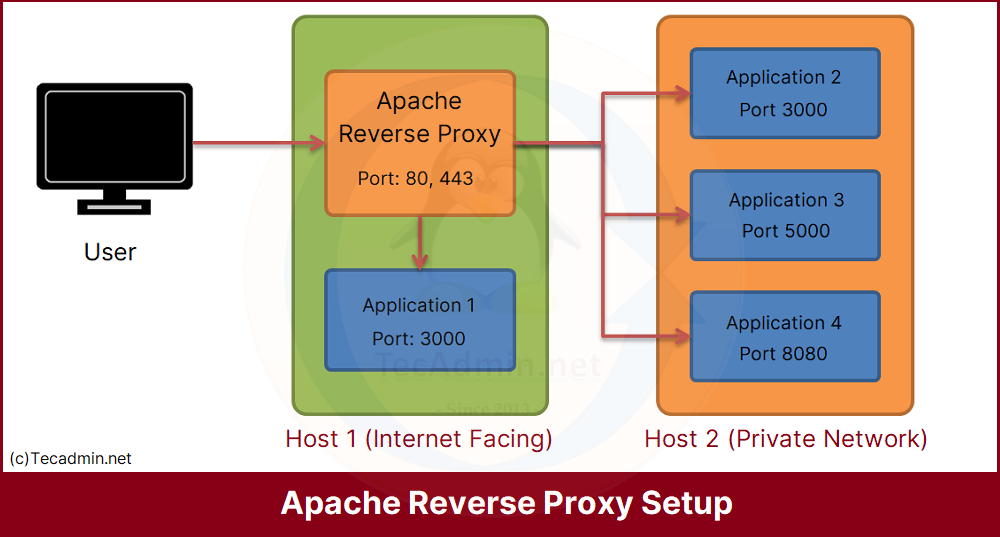
Nginx, a powerful and versatile web server, can effectively handle CORS requests. Its configuration allows you to precisely define which origins, methods, and headers are permitted, ensuring secure and controlled access to your APIs. This detailed guide will walk you through the process of enabling CORS support in Nginx.
Nginx, like Apache, provides a flexible mechanism for implementing CORS. This flexibility translates to granular control over access to your API endpoints. The configuration directives enable precise specification of allowed origins, methods, headers, and other CORS parameters. This level of control is crucial for building robust and secure APIs that comply with CORS best practices.
Nginx Configuration Directives for CORS
The core of CORS implementation in Nginx lies in the use of specific directives within the server block. These directives control the CORS headers returned to the client, enabling or restricting access based on the request’s origin, method, and headers. The `add_header` directive is essential for setting the CORS headers.
Essential Configuration Directives
add_header
: This directive is fundamental for setting the necessary CORS headers in the response. It allows for the specification of various CORS-related headers, such as `Access-Control-Allow-Origin`, `Access-Control-Allow-Methods`, `Access-Control-Allow-Headers`, and more. Properly setting these headers is crucial for allowing or denying access based on the request’s origin, methods, and headers.if
: This directive enables conditional logic in the Nginx configuration. You can use it to determine which requests should receive CORS headers. This is especially valuable when you need different CORS policies for different requests. For example, you can set CORS headers only for requests coming from specific origins.location
: This directive defines the specific URLs or paths for which the CORS configuration should apply. You can use it to create different CORS policies for various parts of your application. For instance, a specific endpoint might require a different set of CORS rules.
Example Nginx Configuration Snippets
Here are a few examples of Nginx configuration snippets demonstrating various CORS use cases:
Allowing CORS for a Specific Origin
“`nginx
location /api
add_header Access-Control-Allow-Origin ‘https://example.com’;
add_header Access-Control-Allow-Methods ‘GET, POST, PUT, DELETE’;
add_header Access-Control-Allow-Headers ‘Content-Type, Authorization’;
“`
This snippet configures CORS for the `/api` path. It allows requests from `https://example.com` using the GET, POST, PUT, and DELETE methods, and specifies that the `Content-Type` and `Authorization` headers are allowed.
Allowing CORS for Multiple Origins
“`nginx
location /api
if ($http_origin = ‘https://example.com’
or $http_origin = ‘https://another-example.com’)
add_header Access-Control-Allow-Origin $http_origin;
add_header Access-Control-Allow-Methods ‘GET, POST’;
add_header Access-Control-Allow-Headers ‘Content-Type, X-Custom-Header’;
“`
This example allows CORS requests from both `https://example.com` and `https://another-example.com` using the GET and POST methods, and specifies allowed headers. Note the use of the `if` directive for conditional logic.
Handling Pre-flight Requests
“`nginx
location /api
add_header Access-Control-Allow-Origin $http_origin;
add_header Access-Control-Allow-Methods ‘GET, POST, OPTIONS’;
add_header Access-Control-Allow-Headers ‘Content-Type, Authorization’;
if ($request_method = ‘OPTIONS’)
add_header Access-Control-Max-Age 3600;
add_header Content-Length 0;
return 204;
“`
This example includes handling OPTIONS requests, crucial for pre-flight requests. This snippet addresses potential pre-flight CORS issues.
Comparison of Apache and Nginx Configurations for CORS
Feature | Apache | Nginx |
---|---|---|
CORS Headers | Set using `Header` directives within `.htaccess` or Apache configuration files | Set using `add_header` directives within the Nginx server block |
Origin Handling | Can be complex with `mod_headers` and `RewriteRule` | Directly configurable using `if` and `add_header` |
Pre-flight Requests | Often requires additional modules or custom scripts | Directly handled using `if` and return codes |
Flexibility | Good, but potentially more complex | Very flexible, allowing precise control over CORS policies |
Common CORS Errors and Troubleshooting
CORS, or Cross-Origin Resource Sharing, can be tricky to configure. Mistakes in the configuration of Apache or Nginx can lead to frustrating errors that block requests from different domains. This section details common CORS issues, their causes, and troubleshooting steps to help you identify and resolve them. Understanding these issues is crucial for building robust web applications that handle requests from various origins.
Misconfigured CORS headers are a primary cause of these issues. This often results in requests being blocked due to a mismatch between the allowed origins, methods, or headers defined in the server’s CORS configuration and the requests made by the client. Accurately identifying and resolving these discrepancies is vital for ensuring smooth communication between your application and external clients.
Identifying CORS Error Messages
Incorrect CORS configuration in Apache or Nginx frequently manifests as HTTP errors or blocked requests. Common error messages indicate the nature of the problem. For instance, a browser’s developer tools might display a “CORS error” message along with a detailed description of the specific error. This often includes a clear indication of the header mismatch. Analyzing the error message is the first step in isolating the root cause.
Troubleshooting Steps for CORS Issues
A systematic approach is essential for troubleshooting CORS problems. Start by examining the server’s CORS configuration files in Apache or Nginx. Verify that the `Access-Control-Allow-Origin` header accurately reflects the expected origins. Ensure that the `Access-Control-Allow-Methods` and `Access-Control-Allow-Headers` headers correctly specify the permitted HTTP methods and headers. A mismatch in these values will lead to CORS issues.
Figuring out how to enable CORS in Apache or Nginx can be tricky, but it’s crucial for smooth API interactions. Fortunately, there are plenty of helpful resources out there. Speaking of tricky situations, the San Jose Sharks recently made a roster move, placing William Eklund on injured reserve and recalling Collin Graf here. Hopefully, this recent change will help the team’s performance on the ice, just like implementing CORS correctly will boost your website’s functionality.
Inspecting HTTP Headers
Inspecting the HTTP headers exchanged between the client and server is crucial for pinpointing the issue. Use browser developer tools or a network analysis tool to examine the request and response headers. Specifically look for the `Access-Control-Allow-Origin` header in the response and ensure it matches the origin of the client request. Check for the `Origin` header in the request and compare it with the `Access-Control-Allow-Origin` header in the response.
Using a Debugging Tool for Analysis
Debugging tools can aid in analyzing CORS interactions. These tools capture the request and response details, enabling you to observe the headers exchanged during a CORS exchange. Using a tool like Postman or similar allows you to inspect the request and response headers and compare them against the server’s CORS configuration. This helps in identifying the source of any mismatch and resolving the issue effectively.
Figuring out how to enable CORS in Apache or Nginx can be a bit of a headache, but it’s crucial for smooth API interactions. Often, when dealing with large datasets, we might opt for a “view more” button instead of traditional pagination. This approach, as detailed in this insightful post on why we choose the view more button instead of pagination , can significantly improve user experience, especially for mobile users.
Ultimately, choosing the right method for displaying data directly impacts the efficiency and user-friendliness of your application, even when enabling CORS for backend APIs.
A debugging tool offers a visual representation of the request and response flow, allowing you to identify the precise point of failure. For instance, you can see if the `Origin` header in the request matches the `Access-Control-Allow-Origin` header in the response. Any mismatch in these headers will directly impact the success or failure of the CORS request.
Advanced CORS Configurations
CORS, or Cross-Origin Resource Sharing, is crucial for enabling communication between different domains. Beyond the basic setup, advanced configurations are necessary for more complex interactions, such as handling preflight requests, custom headers, and diverse access control lists. This section dives into these intricacies, providing practical examples to solidify your understanding.
Understanding the nuances of CORS is essential for building robust and secure APIs that allow external applications to interact with your backend resources. These advanced configurations ensure seamless communication, while adhering to security best practices.
Preflight Requests
Preflight requests are a critical component of CORS. They act as a preliminary step for certain HTTP methods (e.g., POST, PUT, DELETE) that might not be supported by the origin server without prior notification. The browser sends an OPTIONS request to the server to verify if the requested operation is permissible.
The server responds with an `Access-Control-Allow-Methods` header detailing the acceptable methods. This ensures the browser knows whether the actual request is safe to execute. A typical scenario involves a web application interacting with an API to upload data; the preflight request checks if the API allows uploads.
Custom Headers
Custom headers are often used to transmit additional data during cross-origin requests. These headers are crucial for applications that require fine-grained control over data exchange. For example, authentication tokens, specific metadata, or application-specific parameters can be passed through custom headers.
Configuring CORS for custom headers involves including them in the `Access-Control-Expose-Headers` response header. This informs the browser which headers are accessible to the client. This approach is essential for securely transmitting and receiving specific data types or information within the request.
API Endpoint Specific CORS
Different API endpoints might require distinct CORS configurations. This flexibility is crucial for managing access based on the resource being accessed. For example, a user-creation endpoint might have different permissions compared to an endpoint retrieving user profiles.
This involves creating separate CORS rules for each endpoint to grant appropriate access and control. This targeted approach ensures fine-grained security and prevents unintended access to specific data.
Access Control Lists (ACLs)
Access Control Lists (ACLs) define the allowed origins and methods for CORS requests. These ACLs can be quite granular, enabling selective access based on domain, protocol, and port. This granular control ensures only authorized clients can interact with the API.
A well-defined ACL ensures security and restricts access to sensitive data, maintaining the integrity and privacy of the system.
Handling Credentials
Handling credentials is critical when sensitive data is exchanged between origins. The `Access-Control-Allow-Credentials` header is essential for allowing cookies and other authentication credentials to be transmitted. This header is crucial when authentication mechanisms like session cookies are used.
Enabling credentials in the CORS configuration ensures that the client can use cookies for authentication, which is critical for applications requiring secure interactions.
Handling Different HTTP Methods
CORS allows you to configure the permissible HTTP methods (GET, POST, PUT, DELETE, etc.). The `Access-Control-Allow-Methods` header defines which methods are supported by the server for cross-origin requests.
This configuration is vital for controlling the actions allowed on the API. Specifying which HTTP methods are permitted and which are denied maintains the security and integrity of the system.
Figuring out how to enable CORS on Apache or Nginx can be tricky, but it’s a crucial step for web apps. Recently, I’ve been researching this topic, and I’ve stumbled across some fascinating details about Joseph Schaefers in Cupertino, CA, joseph schaefers cupertino ca. His work in the tech industry seems to highlight the importance of secure APIs, which directly connects to the need for properly configured CORS headers.
Getting CORS right is key to ensuring smooth communication between your front-end and back-end.
Security Considerations
Improperly configured CORS can create significant security vulnerabilities, exposing your web application to attacks. Understanding the potential risks and implementing robust security measures is crucial for protecting sensitive data and maintaining user trust. This section details the security implications of misconfigured CORS, how malicious actors can exploit vulnerabilities, and best practices for securing your Apache and Nginx configurations.
Misconfigured CORS policies can lead to cross-site scripting (XSS) attacks, cross-site request forgery (CSRF) attacks, and unauthorized access to protected resources. A common scenario involves an attacker exploiting a poorly defined CORS policy to gain access to data that should be restricted to authorized clients. For example, an attacker might craft a malicious website to exploit a vulnerable CORS policy and obtain sensitive information from your application.
Security Implications of Misconfigured CORS
Misconfigured CORS policies can allow unauthorized access to sensitive data and resources. A malicious actor can exploit these vulnerabilities to steal data, perform unauthorized actions, or inject malicious scripts. This can compromise user accounts, expose confidential information, and disrupt service availability. The severity of the security implications depends on the data being protected and the nature of the attack.
Vulnerability Exploitation by Malicious Actors
Malicious actors can exploit vulnerabilities in CORS implementations to gain unauthorized access. For example, they might create a fake website that mimics a legitimate application and exploit the CORS policy to access sensitive data. This could involve sending crafted requests to the server to bypass security measures and gain access to restricted resources. They can also leverage existing vulnerabilities in the client-side application or browser.
Security Best Practices for Apache and Nginx
Implementing secure CORS policies in Apache and Nginx requires careful consideration of the origins allowed, the HTTP methods permitted, and the headers that can be accessed. A critical aspect is to restrict access to only trusted origins. A comprehensive policy should define which origins are permitted to interact with the server and limit the allowed HTTP methods to prevent unauthorized actions.
- Restrict Origins: Define a whitelist of permitted origins instead of using wildcard ‘*’ characters. This limits access to only trusted domains and prevents attacks from arbitrary sources.
- Specify Allowed Methods: Explicitly list the HTTP methods (GET, POST, PUT, DELETE, etc.) allowed for each origin. Restricting the methods prevents attackers from performing actions they are not authorized to do.
- Control Access to Headers: Specify which headers are allowed in the requests. This limits the information that can be transmitted between the client and server, minimizing potential vulnerabilities.
- Implement Strict Same-Origin Policy: Enforce the same-origin policy as strictly as possible. This helps prevent cross-site scripting (XSS) attacks, as the server is more selective about which resources are accessible from different origins.
- Regularly Review and Update Policies: CORS policies should be reviewed and updated regularly to address any emerging threats or changes in your application’s architecture. This helps to maintain a strong security posture and adapt to new threats.
Mitigation of Potential Risks
To mitigate potential risks associated with misconfigured CORS, a systematic approach is needed. A step-by-step approach can help in identifying and addressing vulnerabilities.
- Identify Sensitive Resources: Determine which resources require protection and define the permissible origins and methods for each.
- Define a Strict CORS Policy: Create a policy that restricts access to only trusted origins and methods.
- Implement Access Control Lists (ACLs): Use ACLs to control access to specific resources and limit the actions that can be performed by different origins.
- Regularly Audit and Test: Conduct regular audits and penetration tests to identify potential vulnerabilities in the CORS implementation.
- Use a Security Scanner: Utilize security scanners to identify potential misconfigurations and vulnerabilities in the CORS policy.
Comparison of CORS Implementation Security Features
Feature | Apache | Nginx |
---|---|---|
Origin Restriction | Highly configurable using mod_headers or .htaccess | Highly configurable using directives like `add_header` |
Method Restriction | Configurable using mod_headers or .htaccess | Configurable using `add_header` and `if` statements |
Header Restriction | Configurable using mod_headers or .htaccess | Configurable using `add_header` and `if` statements |
Same-Origin Policy Enforcement | Inherent in Apache’s configuration | Inherent in Nginx’s configuration |
Practical Examples and Use Cases
CORS, or Cross-Origin Resource Sharing, is crucial for modern web applications, especially those with a front-end and back-end separated by different domains. This separation allows for better organization and scalability, but requires a mechanism to enable secure communication between the two. Practical examples demonstrate how CORS enables this communication and safeguards against security risks.
Understanding the need for CORS in scenarios where front-end applications need to interact with back-end APIs is vital. This interaction often involves fetching data, sending requests, and performing updates. Without CORS, these interactions could be blocked due to security policies. Therefore, CORS implementations are vital for the smooth operation of such applications.
Real-World Application Design Examples
Front-end applications frequently use APIs hosted on different domains. A typical example involves a mobile app (front-end) communicating with a server (back-end) for user authentication or data retrieval. A robust e-commerce platform could use a client-side application (front-end) to fetch product details from a dedicated API (back-end). These examples highlight the necessity of CORS to allow secure communication between these components.
CORS and Front-End/Back-End API Communication
Consider a scenario where a single-page application (SPA) hosted on `https://myapp.example.com` needs to access data from a REST API hosted on `https://api.example.com`. Without CORS enabled, the browser will block the requests from the SPA to the API, preventing the application from functioning properly. CORS provides the necessary mechanism to allow the SPA to communicate with the API securely and efficiently.
Simple REST API with CORS Enabled (Apache)
To illustrate, let’s create a simple REST API using Python’s Flask framework, which is configured with Apache to handle CORS requests.
“`python
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(‘/data’, methods=[‘GET’])
def get_data():
# …API logic to fetch data…
data = ‘message’: ‘Data fetched successfully’
return jsonify(data)
if __name__ == ‘__main__’:
app.run(debug=True)
“`
On the Apache side, you’d need to configure an appropriate .htaccess file or equivalent to add CORS headers to the responses. This configuration ensures that the response will allow requests from specific origins, thus satisfying the CORS requirements.
Simple REST API with CORS Enabled (Nginx)
A similar REST API, implemented with Nginx, would require configuring Nginx to add CORS headers to the responses. This involves setting specific HTTP headers within the Nginx configuration file.
“`nginx
location /api
proxy_pass http://127.0.0.1:5000;
proxy_set_header Host $host;
add_header Access-Control-Allow-Origin ‘https://myapp.example.com’;
add_header Access-Control-Allow-Methods ‘GET, POST, OPTIONS’;
add_header Access-Control-Allow-Headers ‘Content-Type, Authorization’;
“`
This configuration explicitly states that the origin `https://myapp.example.com` is allowed to access the API.
Impact of CORS on HTTP Methods
Different HTTP methods, like GET, POST, PUT, and DELETE, require specific handling in CORS configurations. The server must explicitly allow these methods in the `Access-Control-Allow-Methods` header. For instance, a `POST` request to update data requires the server to allow the `POST` method in the header.
CORS and Performance/Efficiency, Enable cors apache nginx
CORS adds a layer of overhead due to the extra steps involved in handling the preflight requests. However, this overhead is generally minimal compared to the benefits of enhanced security and the ability to support diverse web application architectures. In modern web applications, the performance impact of CORS is often negligible, and the security benefits outweigh any minor efficiency concerns.
Final Thoughts
In conclusion, implementing CORS on Apache and Nginx is a vital step for any web application that needs to exchange data between different domains. By carefully configuring CORS parameters, and adhering to security best practices, developers can ensure secure and efficient communication between their front-end and back-end systems. The detailed examples and troubleshooting tips provided in this guide will equip you with the necessary knowledge to confidently configure and manage CORS in your applications.